Neo4j Java Object Graph Mapper 1.1.0 Released
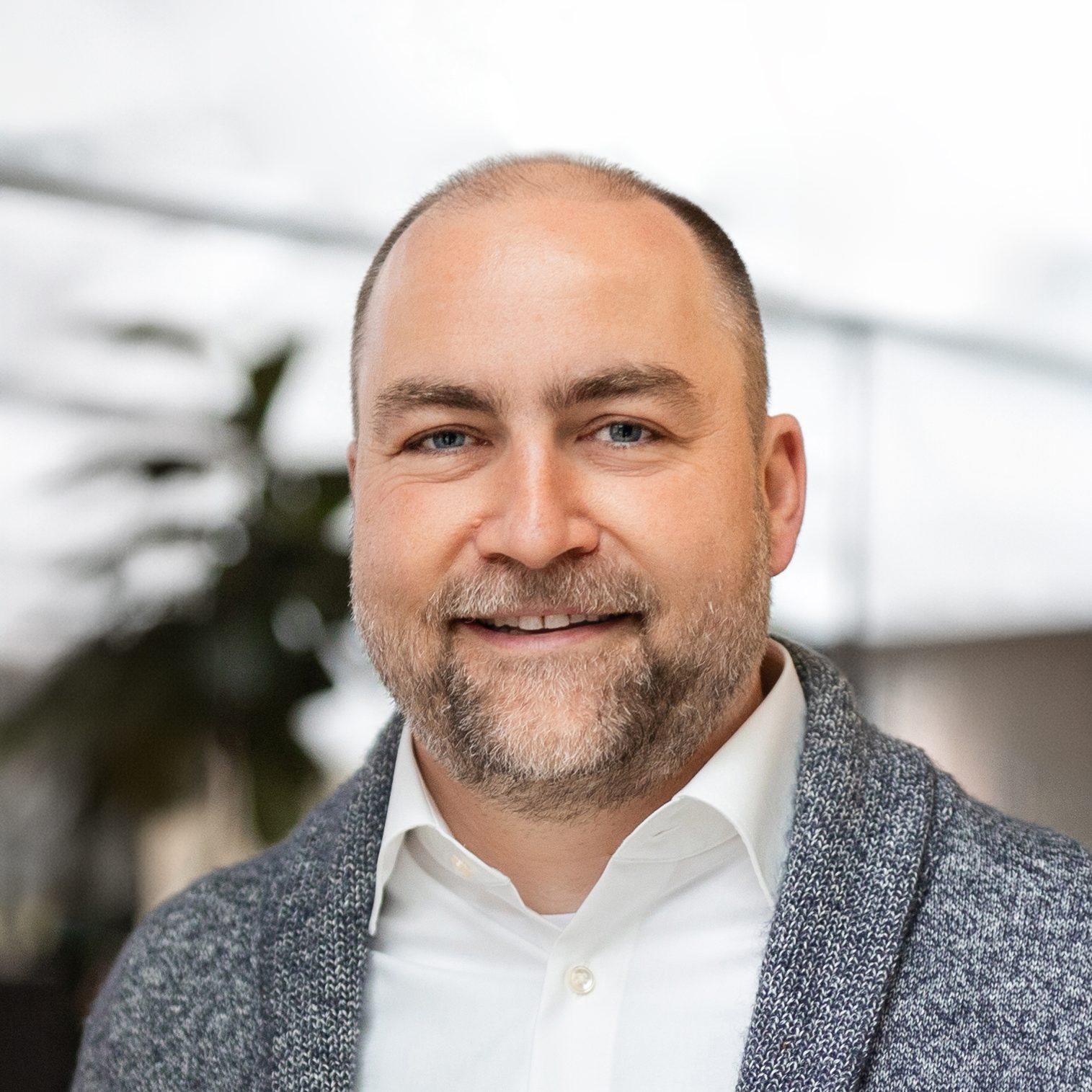
Head of Product Innovation & Developer Strategy, Neo4j
3 min read
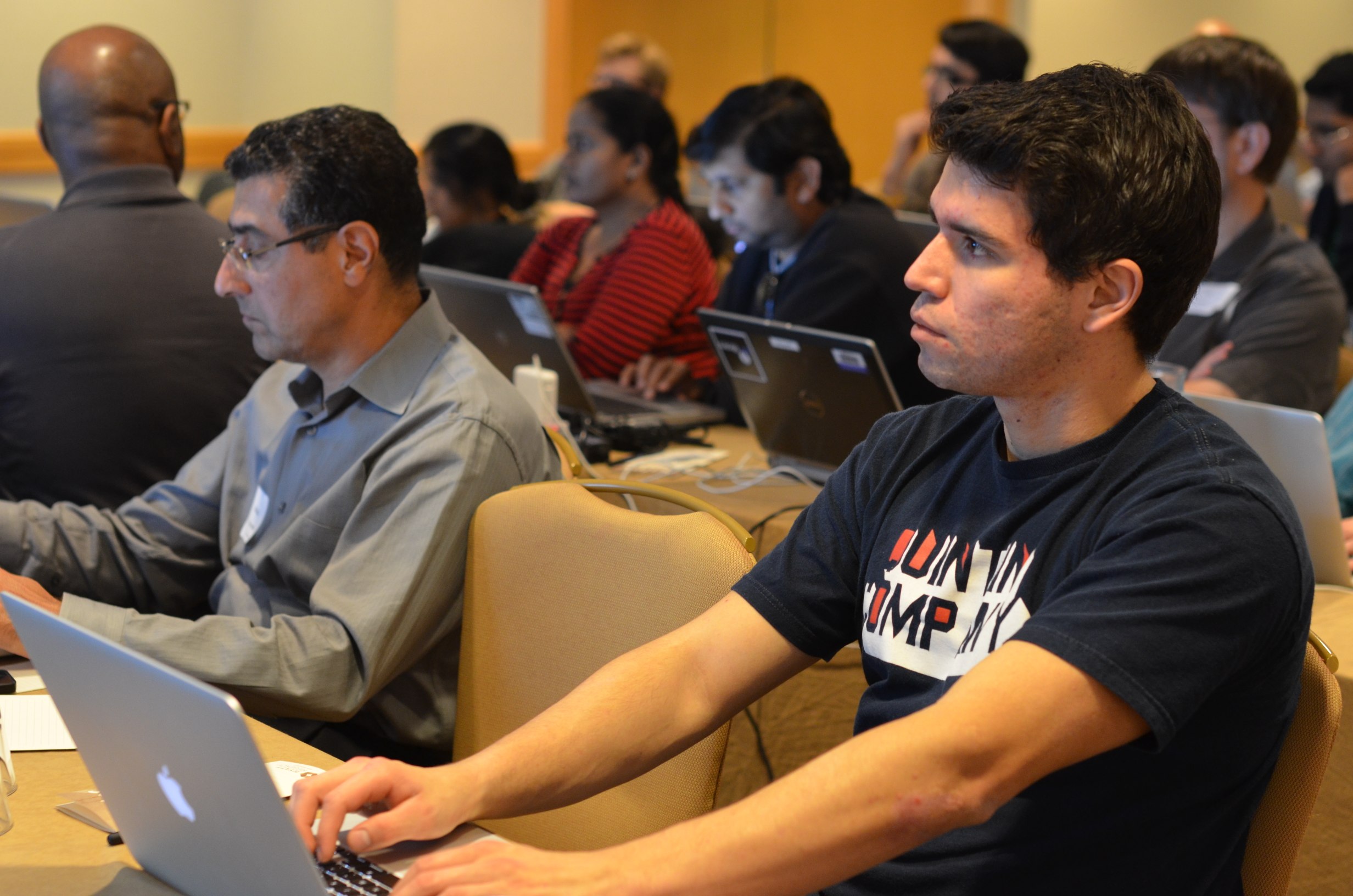
Many of you might be aware of the effort, we’re putting behind the Spring Data Neo4j 4.0
development with our partner GraphAware.
What you may not know, is that we learned from the past and separated the Object Graph Mapping functionality from the Spring Data project.
Today we want to publicly announce the availability of version 1.1.0 of this library which allows you to use Object Graph Mapping from any Java or JVM-based application with no other dependency.
The core of the mapping, querying and conversion facilities live in an officially supported project and library called Neo4j-OGM which you can find on GitHub permissively licensed under Apache License v2.0.
Background & Features
This library was written completely from scratch with special focus on performance. For now, it’s focused on working with the Neo4j server and using Cypher and the transactional Cypher endpoint to interact with Neo4j.
That translates into high-performance transactional operations over the wire.
Other features include:
- Fast byte code scanning for gathering the mapping metadata
- Tunable read and write depths for immediate loading and updating of the neighborhood of an entity
- Session-based delta tracking so only minimal updates are send to Neo4j
- Simple configuration
- Support for auth and Neo4j 2.2.x and 2.3.x
Some notable differences to other OGMs and Spring Data Neo4j 3.x include:
- Indexing is a concern outside of the application
- Other Neo4j REST endpoints are not supported
Setup & Configuration
Just add this library to your dependency declaration and you’re good to go:
<dependency>
<groupId>org.neo4j</groupId>
<artifactId>neo4j-ogm</artifactId>
<version>1.1.0</version>
</dependency>
If you want to use the library in your project you can do so by setting up the dependencies and this configuration
public class Neo4jSessionFactory {
private final static SessionFactory sessionFactory =
new SessionFactory("school.domain"); (1)
private static Neo4jSessionFactory factory = new Neo4jSessionFactory();
public static Neo4jSessionFactory getInstance() {
return factory;
}
public Session getNeo4jSession() {
return sessionFactory.openSession(System.getenv("NEO4J_URL")); (2)
}
}
1 | Session factory with base domain package |
2 | Neo4j Connection, here a remote server |
Entities & Services
For your entities, you can choose to annotate them or not. By default, (though overridable with annotations) it uses the class name for labels and field names for properties and relationship types.
An entity looks like this:
public class Student {
private Long id;
private String name;
@Relationship(type = "ENROLLED")
private Set<Enrollment> enrollments;
private Set<Course> courses;
@Relationship(type = "BUDDY", direction = Relationship.INCOMING)
private Set<StudyBuddy> studyBuddies;
}
And a concrete service to provide persistence:
public class StudentService implements Service<Student> {
private static final int DEPTH_LIST = 0;
private static final int DEPTH_ENTITY = 1;
private Session session = Neo4jSessionFactory.getInstance().getNeo4jSession();
public Iterable<Student> findAll() {
return session.loadAll(Student.class, DEPTH_LIST);
}
public Student find(Long id) {
return session.load(Student.class, id, DEPTH_ENTITY);
}
public void delete(Long id) {
session.delete(session.load(Student.class, id));
}
public Student createOrUpdate(Student entity) {
session.save(entity, DEPTH_ENTITY);
return find(student.getId());
}
}
Feedback
Find more details on how to use the Java Object Graph Mapper in the online documentation and the example project.
Please provide us with feedback and ideas by testing it out, raising GitHub issues or via email.
Happy mapping!
–Michael
References
- Neo4j-OGM on GitHub
- Online Documentation
- Example University Angular + Neo4j OGM Application
- GitHub issues
Looking to learn more about graph databases? Click below to get your free copy of O’Reilly’s Graph Databases and learn everything you need to know about graph technologies and Neo4j.