Run Cypher From Java
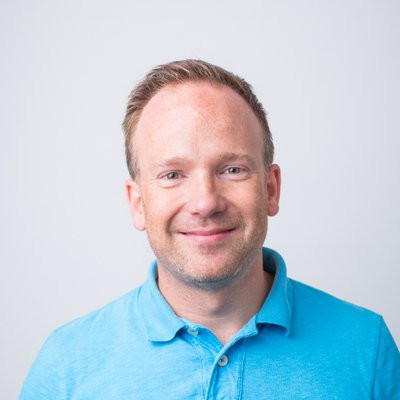
Technical Curriculum Developer, Neo4j
3 min read
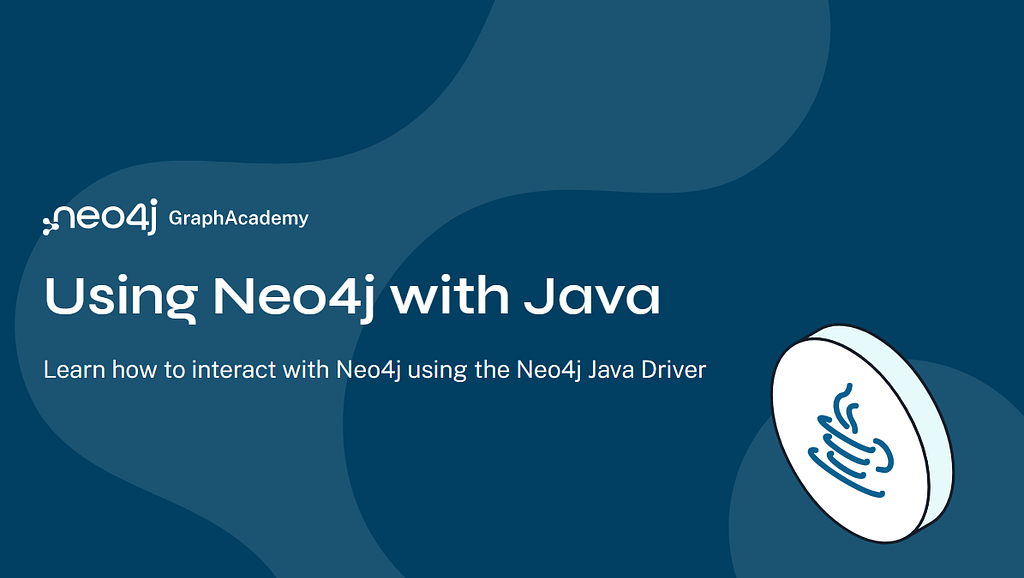
Are you a Java developer looking to take advantage of graphs and Neo4j?
There’s a new GraphAcademy course called Using Neo4j with Java, where you can learn how to integrate the Neo4j Java driver into your Java application.
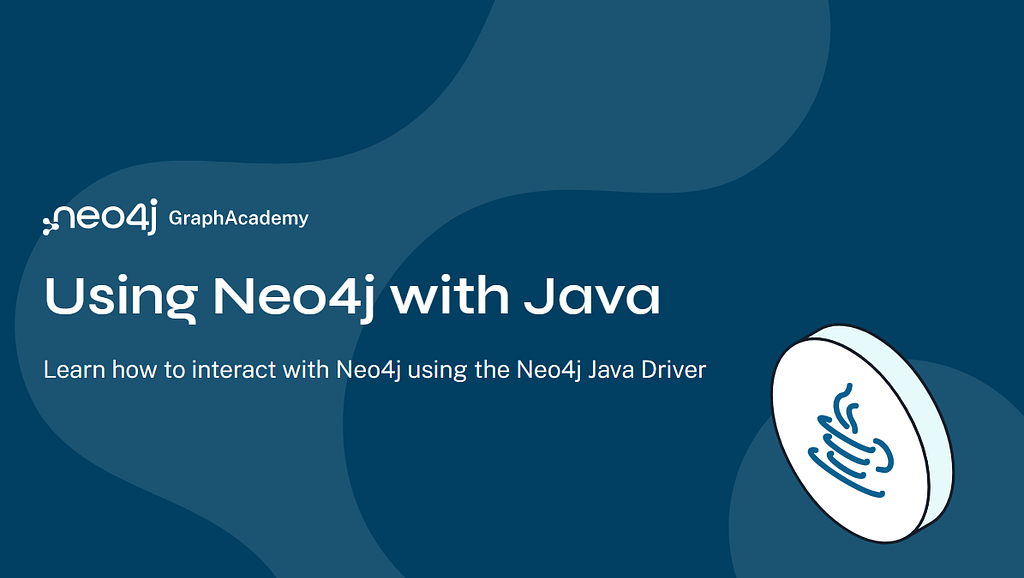
The most common thing you will need to do when using Neo4j with Java is run a Cypher query and parse the results. The process is really simple:
- Import the driver
- Connect to a server
- Verify the connection
- Execute a Cypher query
- Parse the results
- Close the connection
Import the Driver
The driver is distributed via Maven, you can find snippets and example POM files in the Maven Central Repository — neo4j-java-driver package.
You’ll need to import the GraphDatabase and AuthTokens classes from the Neo4j driver package:
import org.neo4j.driver.GraphDatabase;
import org.neo4j.driver.AuthTokens;
public class App {
public static void main(String[] args) {
String NEO4J_URI = "bolt://localhost:7687";
String NEO4J_USERNAME = "neo4j";
String NEO4J_PASSWORD = "mypassword";
// Create a new Neo4j driver instance
// Verify the connection
// Execute a Cypher query
// Parse the results
// Close the connection
}
}
Connect to a Server
Create an instance of the GraphDatabase.driverclass, passing your credentials:
// Create a new Neo4j driver instance
var driver = GraphDatabase.driver(
NEO4J_URI,
AuthTokens.basic(
NEO4J_USERNAME,
NEO4J_PASSWORD)
);
Verify the Connection
You can test the connection by calling the verifyConnectivity method:
// Verify the connection
driver.verifyConnectivity();
The driver will raise an exception if the connection cannot be made.
Execute a Cypher Query
The executableQuery method executes a Cypher query and returns the results:
// Execute a Cypher query
var result = driver.executableQuery(
"MATCH (c:Customer) RETURN c.name AS name, c.age AS age"
).execute();
Parse the Results
The executemethod fetches a list of records and loads them into memory:
// Parse the results
var records = result.records();
records.forEach(r -> {
System.out.println(r.get("name"));
System.out.println(r.get("age"));
});
You can iterate through the records and use get to retrieve return values.
Close the Connection
Once you finish with the driver, call close to release any resources:
// Close the connection
driver.close();
Here’s the complete code that makes a connection, executes a query, and parses the results:
import org.neo4j.driver.GraphDatabase;
import org.neo4j.driver.AuthTokens;
public class App {
public static void main(String[] args) {
String NEO4J_URI = "bolt://localhost:7687";
String NEO4J_USERNAME = "neo4j";
String NEO4J_PASSWORD = "mypassword";
// Create a new Neo4j driver instance
var driver = GraphDatabase.driver(
NEO4J_URI,
AuthTokens.basic(
NEO4J_USERNAME,
NEO4J_PASSWORD)
);
// Verify the connection
driver.verifyConnectivity();
// Execute a Cypher query
var result = driver.executableQuery(
"MATCH (c:Customer) RETURN c.name AS name, c.age AS age"
).execute();
// Parse the results
var records = result.records();
records.forEach(r -> {
System.out.println(r.get("name"));
System.out.println(r.get("age"));
});
// Close the connection
driver.close();
}
}
Learn More
You can learn more about how to use the Neo4j Java driver in the Using Neo4j with Java course on GraphAcademy.
The course will get you started developing Java applications with Neo4j and covers topics such as passing parameters to queries, dealing with graph data types, and managing transactions.
What Is Neo4j GraphAcademy?
Neo4j GraphAcademy offers a variety of courses completely free of charge, teaching everything from Neo4j Fundamentals to Building Knowledge Graphs With LLMs and much more.
Run Cypher From Java was originally published in Neo4j Developer Blog on Medium, where people are continuing the conversation by highlighting and responding to this story.