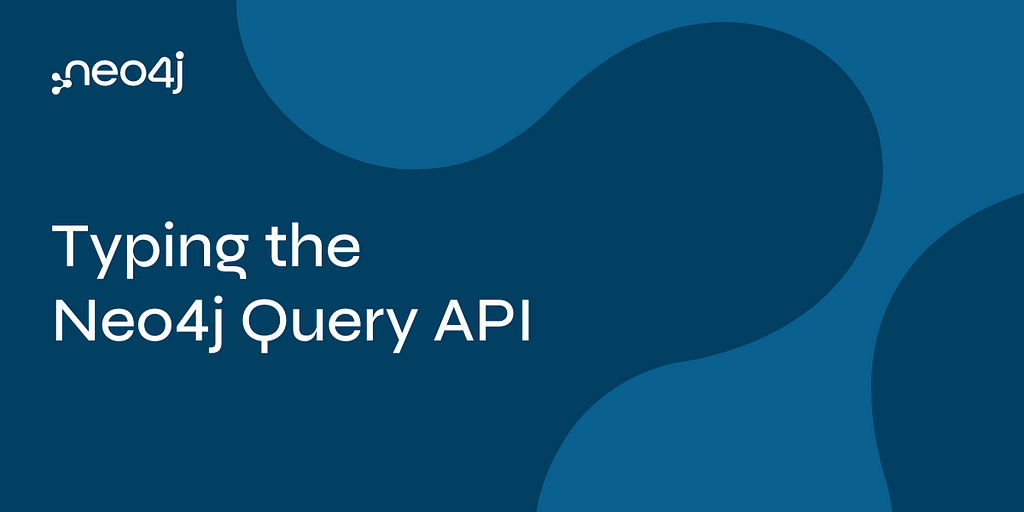
Typing the Neo4j Query API
Jul 23 8 mins read
Discover a simple way to query Neo4j through your favorite HTTP client with Neo4j Query API. Read more →
Discover a simple way to query Neo4j through your favorite HTTP client with Neo4j Query API. Read more →
Learn a low-code approach to combine Neo4j Bloom graph visualization with OTX to enable powerful visual threat investigations. Read more →
The Neo4j GenAI Package for Python equips you with the tools to efficiently manage retrieval and generation processes in a RAG setup. Read more →
Learn how to use unstructured.io for PDF document parsing, extracting, and ingestion into the Neo4j graph database for GenAI applications. Read more →
Chemical reactions are represented visually as a graph. Let's explore them in the Neo4j Graph Database. Read more →
We’re pleased to announce the launch of a new Cypher co-pilot available in Neo4j Aura to all Workspace users. Read more →
Learn how to combine text extraction, network analysis, and LLM prompting and summarization for improved RAG accuracy. Read more →
We’ve decided to restrict all new third-party Graph App installations. Learn what Graph Apps are affected and what options are still available. Read more →
Learn how to build a GraphRAG application almost entirely in Cypher, using knowledge graphs and vector search in Neo4j. Read more →
Learn the mix and batch technique to parallelize your relationship loading in Neo4j two to three times faster. Read more →
Learn how to transfer your MongoDB data and object model to Neo4j and how to use SemSpect to gain insights into your business data. Read more →
Learn how to perform entity deduplication and custom retrieval methods using LlamaIndex to increase GraphRAG accuracy. Read more →
From SQL to GQL, the new graph query language standard and a pivotal development for advancing data analytics in the era of GenAI and beyond. Read more →
Extract and use knowledge graphs in your GenAI applications with the LLM Knowledge Graph Builder in just five minutes. Read more →
The Neo4j GraphRAG Ecosystem Tools make it easy to develop GenAI applications grounded with knowledge graphs. Read more →
Neo4j, LLM creators, RAG orchestrators, knowledge graph designers, researchers, and deep thinkers gathered in San Francisco Presidio to explore GenAI. Read more →
Learn how to ingest data from relational data into your Neo4j graph database automatically, by using the Neo4j Runway Python Library. Read more →
Learn how to explore and ingest your relational data into a Neo4j graph database in minutes with the Neo4j Runway Python Library. Read more →
Discover how Neo4j and chatbots transform ASVS accessibility, enhancing application security with graphs and AI-driven insights. Read more →
Photo by Growtika on UnsplashThere are so many options when it comes to languages, frameworks, and tools for building generative AI (GenAI) applications. When you are just getting started, these decisions and figuring out how to integrate everything can be overwhelming.My… Read more →
Create sophisticated solutions that understand and process natural language with unparalleled efficiency and accuracy using the LlamaIndex library with the Neo4j graph database. Read more →
Learn how we build a context-rich chatbot to answer Mahabharata questions using knowledge graphs and retrieval-augmented generation (RAG). Read more →
Learn how graph and vector search systems can work together to improve retrieval-augmented generation (RAG) systems. Read more →
Introducing the Neo4j LangChain Starter Kit for Python developers, which generates GenAI answers backed by data stored in a Neo4j Graph Database. Read more →
Part 2 of analyzing the Mahabharata's connections with knowledge graphs and building a chatbot using Google Gemini to answer questions. Read more →