Neo4j Data Access for Your .NET Core C# Microservice
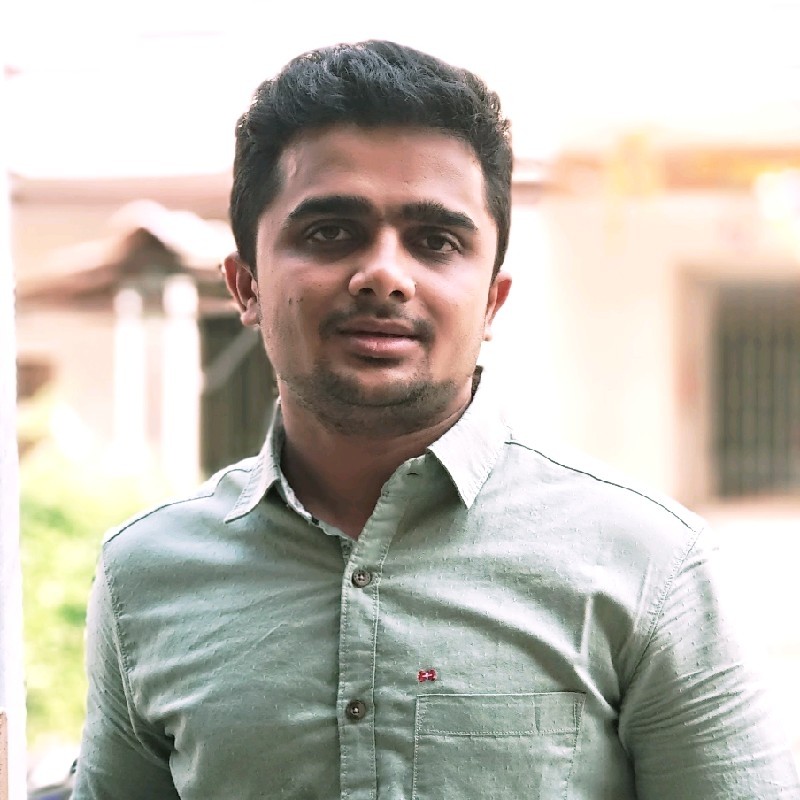
Consulting Engineer, Neo4j
4 min read
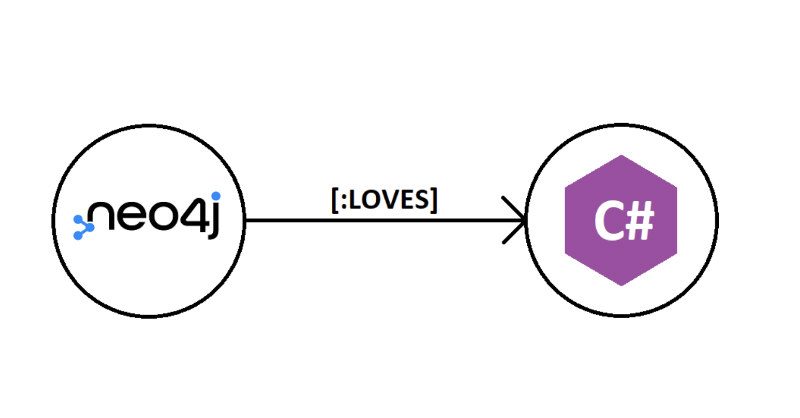
Neo4j is the industry leader and pioneer of graph database technology and is currently the top-rated graph database management system in the world.
In my experience so far with designing solutions and applications around Neo4j, I came across many materials and online resources from Neo4j and its huge community. There are plenty of resources on Neo4j around various technologies. Neo4j provides excellent drivers for various programming languages to build seamless graph data connectivity with your application.
Drivers & Language Guides – Developer Guides
C# is my first love as a programming language, and Visual Studio development experience will always be my preferred one. I was brought up as a programmer in the .NET and C# world. Hence, I decided to share an approach to effectively use the Neo4j C# driver to develop the data access layer for a .NET Core application.
I am going to share an example of a Neo4j-based Microservice (Web API). However, with out-of-the-box dependency injection (DI) and Service Provider features from .NET Core, this approach can be used for other types of applications, too.
Before you start with .NET Core implementation, I suggest you read a particularly good article by David Allen on Neo4j driver general best practices. These practices apply to all programming languages.
.NET Core Specific Implementation
For developing a Neo4j Data Access for .NET Core, I have divided the approach into the steps you’ll see below.
Have a Neo4j Database Instance Up and Running
If you already have a Neo4j database instance set up and running, you can skip this step. If not, you can easily set up a free Neo4j Aura (Database as a Service) in a hassle-free way. Use the below link to proceed.
Neo4j Aura – Fully Managed Cloud Solution
Alternatively, you can use Neo4j Desktop on your local machine or a Neo4j Sandbox.
While loading the Aura instance or Sandbox, use the prebuilt Movies dataset. If you are using Neo4j Desktop or a plain database, run command :play Movies in your Cypher command bar and follow the data load step to load the test data.
Add the Neo4j Official Driver NuGet Package to Your .NET Core Project
You can install the driver package using the NuGet package manager console or package manager interface in Microsoft Visual Studio. Install this package to the data access project. I suggest using a separate project for data access instead of the main API or application project.
Application Settings
Then go to your app settings file: Neo4j Bolt/Neo4j connection string, Neo4j username, password, and database instance name.
You should implement additional security measures for protecting your Neo4j password instead of specifying it in plain text. For example, try an encryption of your password text, or use some vault to keep your credentials safe.
Startup: Dependency Registration / Dependency Injection for Neo4j Driver
We are injecting the Neo4j Driver instance as a singleton so that we do not have to manage the driver instance on each request.
IAsyncDisposable implementation of Neo4j Data Access Class
IAsyncDisposable interface provides a mechanism for releasing unmanaged resources asynchronously. We injected the Neo4j Data Access class as a scoped dependency. Neo4jData Access Wrapper will establish a database session. We will manage the Neo4j session using IAsyncDisposable.
I have developed the Wrapper methods keeping easy implementation and reusability in mind. You are free to develop your own version of Wrapper methods.
Data Access Interface
Data Access Implementation
Domain Repository Methods for Your Cypher Query and Parameter Prep
You may notice I used the Cypher statement RETURN p{ .name, born: p.born }
in my method to return Person data. Cypher supports a concept called “map projections,” which allows for easily constructing map projections from nodes, relationships, and other map values as shown in the below screenshot.
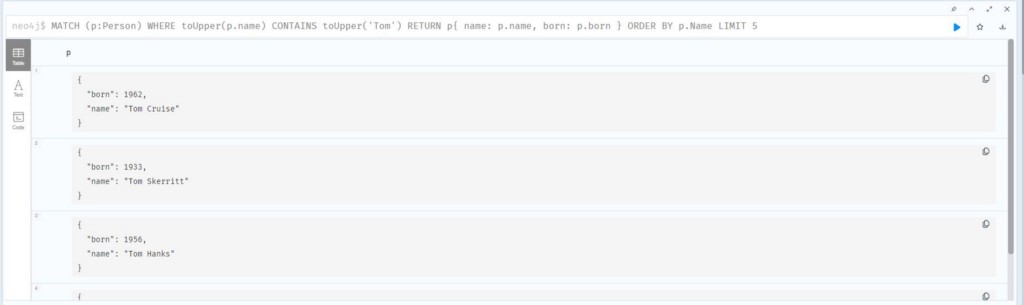
With Cypher projections, I populated List<Dictionary<string, object>>
collection. If your API just needs to provide plain results without additional manipulation, you can simply return this collection. This helps you avoid extra boxing and unboxing. Clients can parse the JSON to their desired object types.
For example, below is the swagger response for my API that returns plain data.
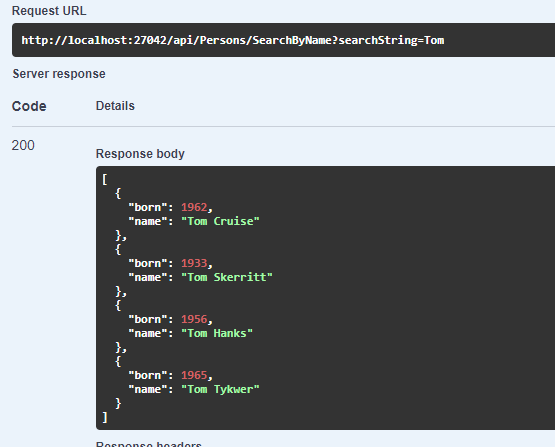
Thanks for spending the time to read this. I hope you find it helpful!
Please share so it reaches more people!
Neo4j Data Access for your Dot Net Core C# Microservice was originally published in Neo4j Developer Blog on Medium, where people are continuing the conversation by highlighting and responding to this story.