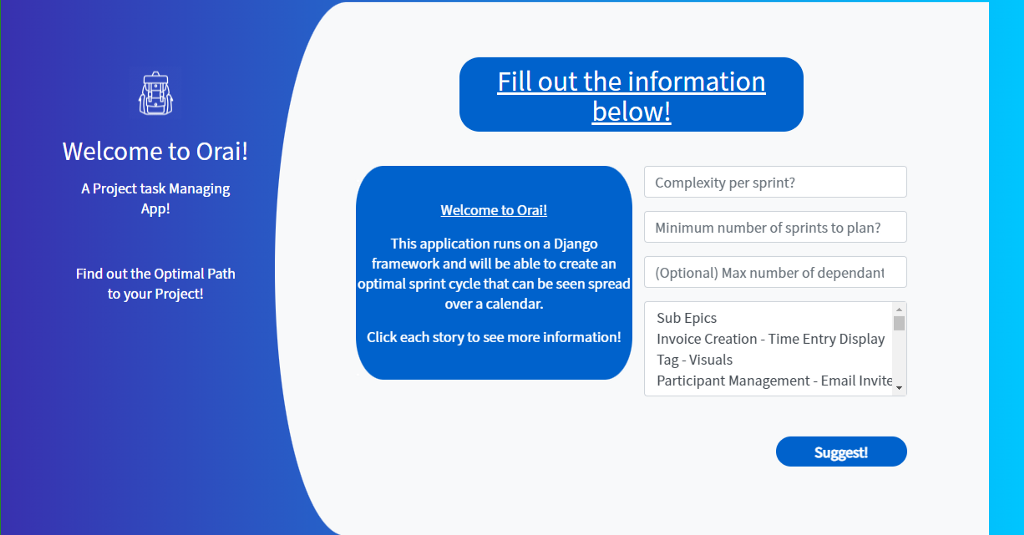
A student project was developed though Florida Atlantic University’s Senior Design Program by student team Logic13.
Background
Sprint cycles are an extremely common way for development teams to plan and execute the development of projects. This agile method can be used by a variety of different development teams. Working in sprint cycles allows teams to get fast feedback, improve their product’s quality, reduce risk, and it makes it easier to stay on schedule.
The Problem
Project Managers must work carefully to make sure the sprint cycles can flow as best as possible with minimal stagnation. To do so, Project Managers set aside time for sprint planning where they can create a road map of what tasks need to be done in what order so that the project can progress and be completed. This can be especially difficult to accomplish since some tasks can be more important or more complicated than others or rely on the completion of other tasks before they may be worked on.
The Solution
The Orai application is a recommendation engine built around project management data. It combines the querying power of the Neo4j graph database with the flexibility of the Django web framework using the django-neomodel plugin.
The Orai system monitors all tasks dependent on each other and builds sprints from them with the highest priority tasks getting scheduled first. The length of these sprints is based on each tasks’ complexity, allowing the user to gain more control of how much the development team can handle.
Furthermore, the user can control the number of sprints, the complexity of sprints, the essential tasks, and the number of essential tasks that can be worked on per sprint.
Implementation Details
Orai takes in four user inputs:
- The max complexity value of each sprint
- Minimum number of sprints desired
- (Optional) The maximum length of the dependency tree
- A set of
Story
s to be prioritized
The Story Model and Relationships are extremely lightweight – the application uses only Story nodes and a REQUIRES
relationship between Story
s:
from neomodel import (
StringProperty,
StructuredNode,
RelationshipTo,
IntegerProperty,
FloatProperty,
BooleanProperty
)
class Story(StructuredNode):
# Properties
nodeID = IntegerProperty(index=True, db_property='id')
sentimentScore = StringProperty()
sentiment = StringProperty()
notes = StringProperty()
name = StringProperty()
value = StringProperty()
acceptance_criteria = StringProperty()
complexity = IntegerProperty()
priority = FloatProperty()
approved = BooleanProperty()
# Relationships
requires = RelationshipTo('.story.Story', 'REQUIRES')
The project used a dataset from The SilverLogic’s internal project management system.
MATCH p=(:Story)-[:REQUIRES]->(:Story)
RETURN p
LIMIT 10
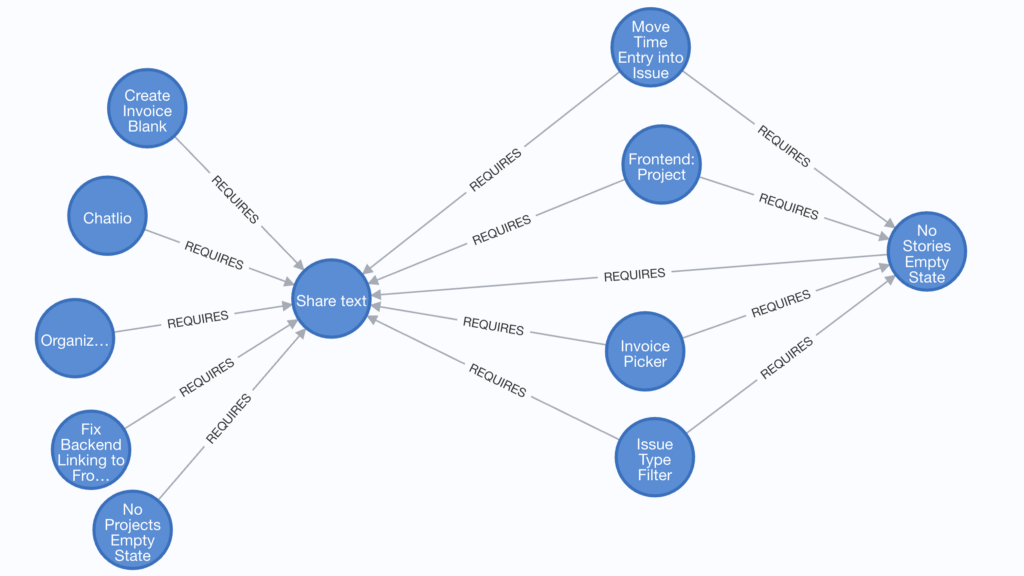
In the example output above, if a team wanted to prioritize the implementation of the Invoice Picker
story, they would have to first complete the Share text
and No Stories Empty State
stories, as they are prerequisites.
Given enough team members and a long-enough sprint, it might be possible to work on Share text
and No Stories Empty State
in parallel, and immediately move to Invoice Picker
once both tasks are completed. If the team knows roughly how many complexity points they can complete in one sprint – and have thoughtfully given their best estimates to the stories in the backlog – having a planning tool that can provide example roadmaps that take into account inter-story relationships can be a useful starting point for planning and scheduling.
Example Input Form
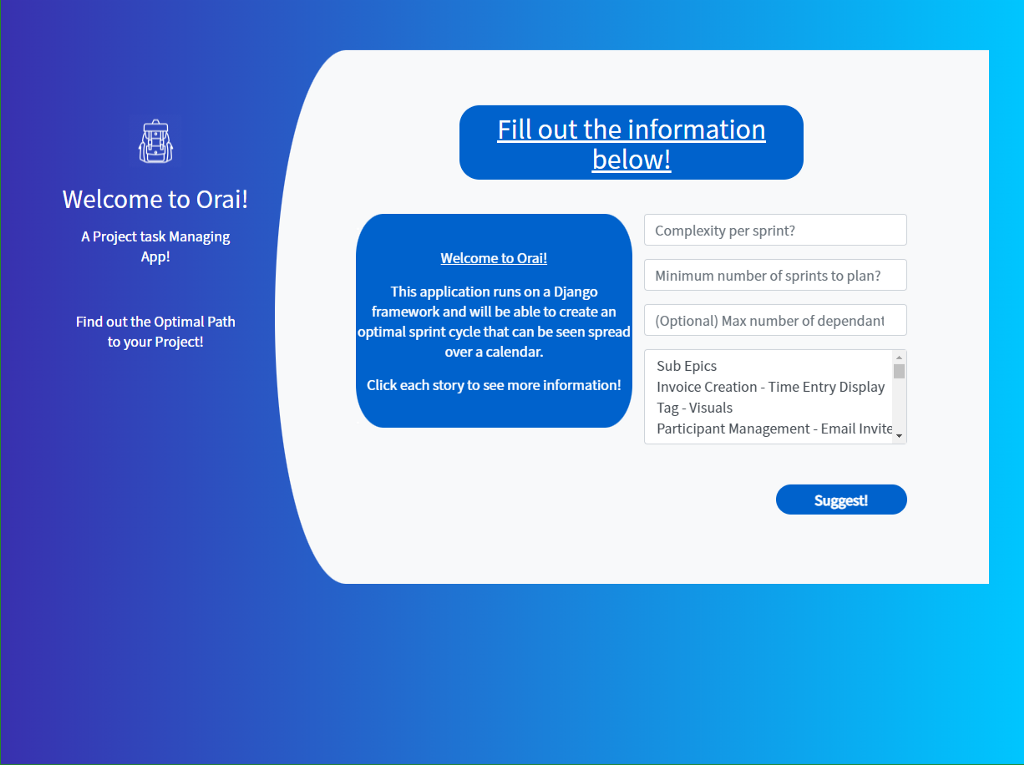
Imagine selecting the following parameters:
Max complexity value per sprint: 12
Minimum number of sprints: 3
Priority
Story :
Epic / Actor / Platform Archiving
- >
Harvest Integration V2 Phase 4 Time Entry Augmentation
Invoice Time Entry Moving
Example Output
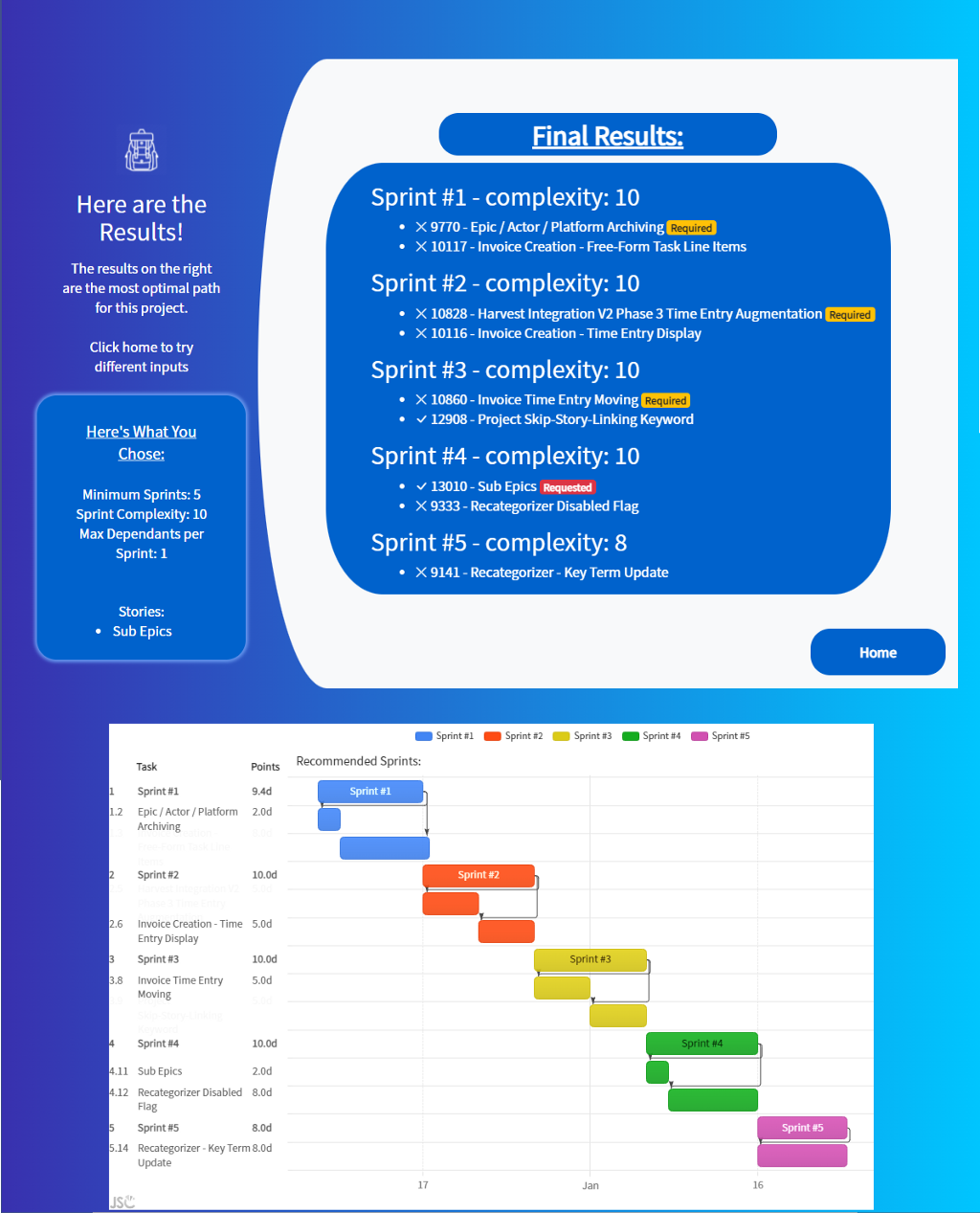
The above is an example of a sprint cycle. The required stories are organized such that the stories it depends on are scheduled before it. For example, Sub Epics
requires that Invoice Time Entry Moving
be completed first.
Conclusion and Retrospective
Over the course of two semesters, Logic13 was able to successfully design and implement a low-cost solution to the project sponsor’s (The SilverLogic) requested problem.
Our project, Orai, is a user-friendly system that can generate optimal sprint cycles from user inputs and display them along with informative details about the sprints and the tasks.
The team was able to learn new programming and computer skills, such as working with and collaborating through GitHub. The team also learned about software development and how to help customers with their requests by giving them alternatives and explanations.
If we had the opportunity to start over and do it again, we’d make a few improvements. One is that on the suggestion page where the user inputs the information, we would add more information to the list of tasks to make it easier for the user to make a choice. This would make the system easier and more convenient to use for the user. Besides that, the team believes this solution is the most optimal and efficient way to schedule tasks for sprints. We are very proud of the work put into the development of the system.
Team Logic13
Team Logic13 (pictured below) consisted of very talented individuals that came together to develop a great system. Felix Medrano took on the role of project lead and handled project scheduling and synchronization, as well as communicating with the sponsor.
Because of his experience with web development and algorithms he would develop the scheduling algorithm used for Orai as well as develop the front end. Valentina Diaz, also having experience with the algorithms we would need, oversaw the chart development and helped with documentation. Because of his experience in the field, Jacob Christensen oversaw the back end and the development of the foundation for the project, including database and system management.
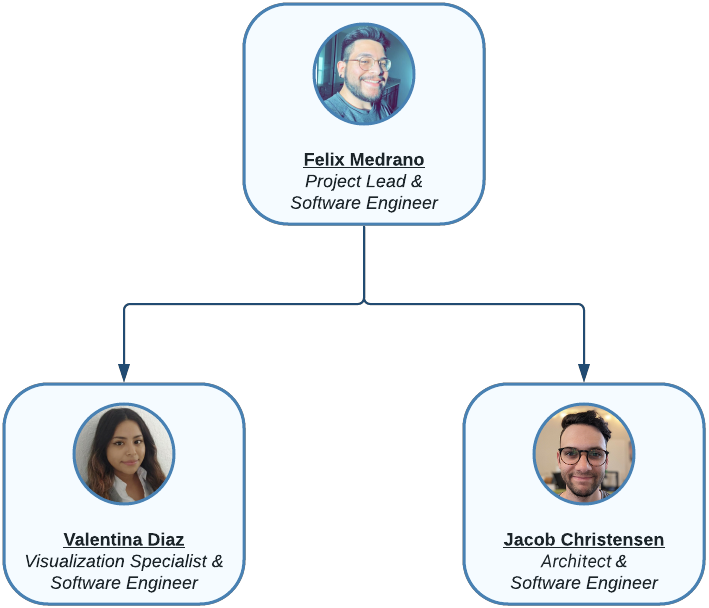
Further Resources
- Fall 2021 Showcase
- GitHub – neo4j-examples/paradise-papers-django: A simple Django web app for searching the Paradise Papers dataset backed by Neo4j
- Future Sponsors
Orai: Project Planning with Neo4j was originally published in Neo4j Developer Blog on Medium, where people are continuing the conversation by highlighting and responding to this story.