We migrated Neo4j’s Graph Examples Portal (AKA the Graph Gist Portal) from Ruby on Rails to GRANDstack (GraphQL, React, Apollo, Neo4j Database). Here is how we did it.
It was an exciting project, as I love the combination of React and GraphQL and a graph database.
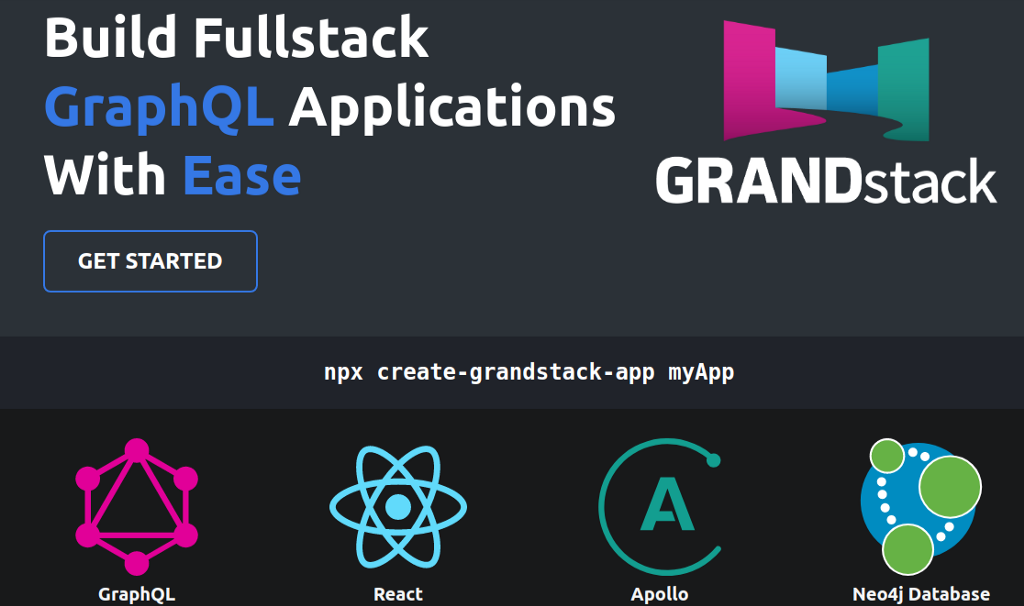
About the Portal
The Portal is a self-serve content management system (CMS) for Neo4j Graph Examples. Graph Examples, also called “GraphGists” are small, self-contained descriptions of graph use cases, including real data setup and queries to demonstrate concepts specific to their domain.
You can find it live and running here at portal.graphgist.org
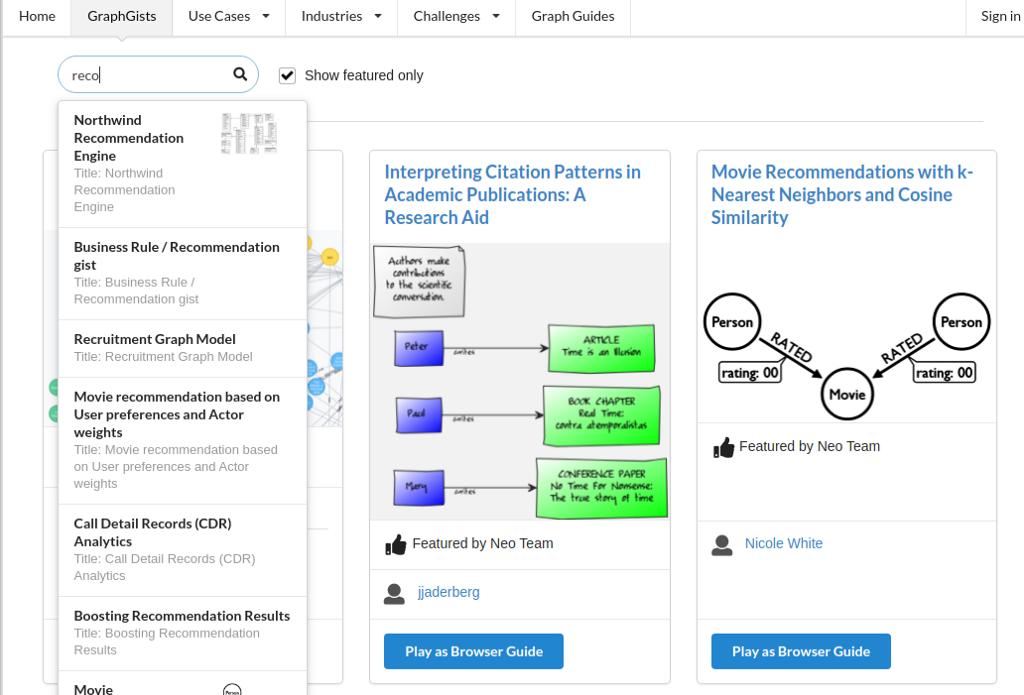
The Architecture
The portal is a relatively lightweight content management system.
Although authors interact with the portal’s web app to upload and edit their content, and the admins use the portal to review and approve the content.
The vast majority of users interact with graph examples either via the Neo4j Desktop Graph App or on Neo4j.com. In short, the majority of readers of the graph examples are not even aware of the portal.
The Domain Model
Nodes:
User : Represents the authenticated users of the portal. They can add and edit content and rate existing content via their Person node.
GraphGist : The published Graph Examples visible on Neo4j.com
GraphGistCandidate : The unapproved content submitted by users.
Use Case , Challenge , Industry : Categories assigned to GraphGist and GraphGistCandidate to be able to place related content into thematic buckets.
Relationships:
IS_PERSON : The association between a User and the Person who writes the content.
WROTE: The association between a Person and their GraphGistCandidate
IS_VERSION : The association between the GraphGistCandidate and the “final” GraphGist
RATES: The relationship between a User and a GraphGist rated by the user
FOR_INDUSTRY, FOR_USE_CASE, FOR_CHALLENGE: The relationship between GraphGist and their thematic category
Example: Graph Examples and Related Use Cases and Industries
Here is an example for a few graph examples in the Sports and Recreation “Industry” and a number of Use Cases.
Summary of Features
Anonymous Users: Consume Content
Anonymous users can:
- View graph examples (the content) on Neo4j Browser as interactive guides, rendered to slide format from the source
- View graph examples on the Neo4j WordPress Site powered by the GraphQL API
- Search and browse graph examples on the portal itself in the React App
- Create accounts and become Authenticated users
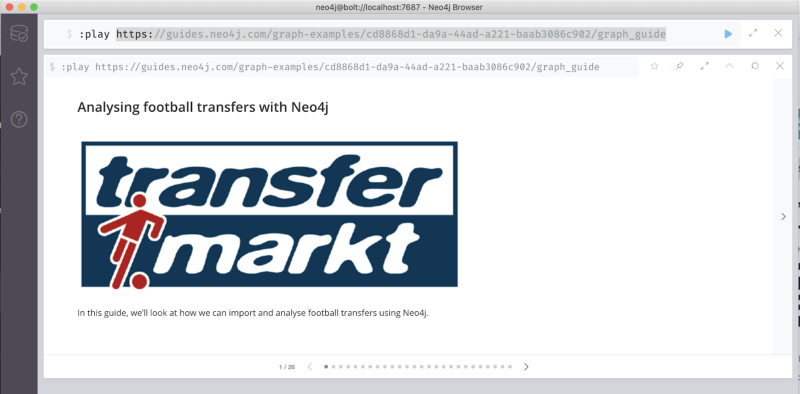
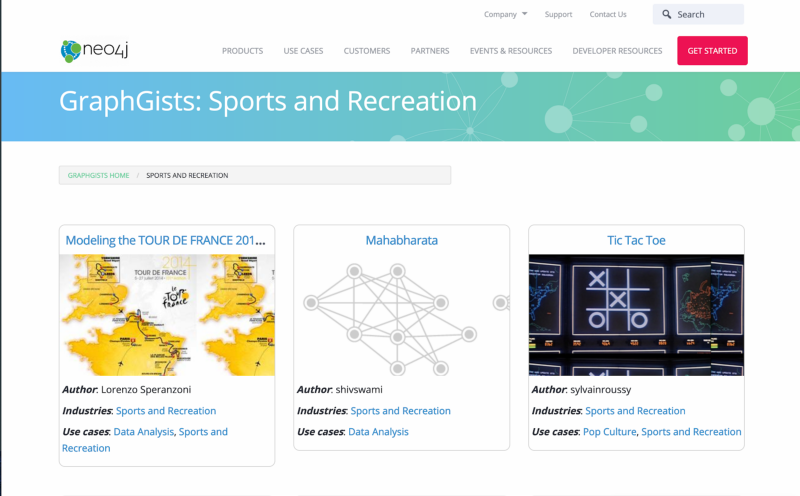
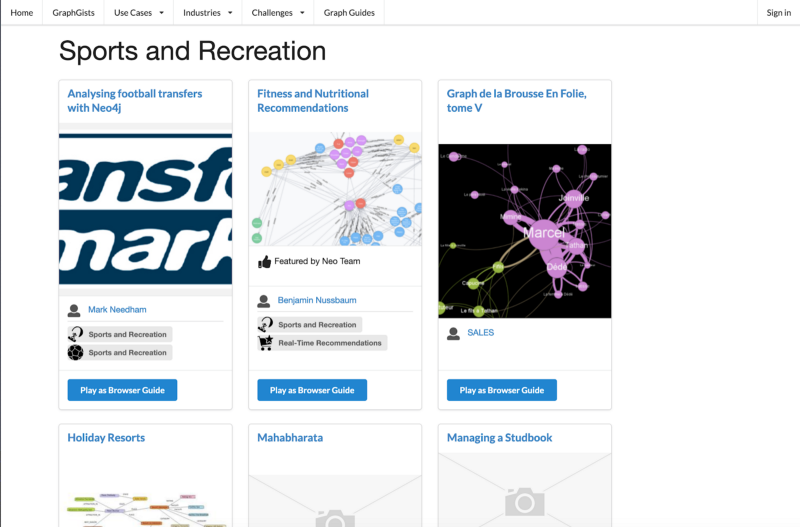
Authenticated Users: Create Content
Authenticated users can:
- Create and edit graph example candidates to preview inline
- Save their work before submitting for approval
- Assign their candidates to industries, use cases, and challenges
- Create new graph example candidate by editing an approved graph example
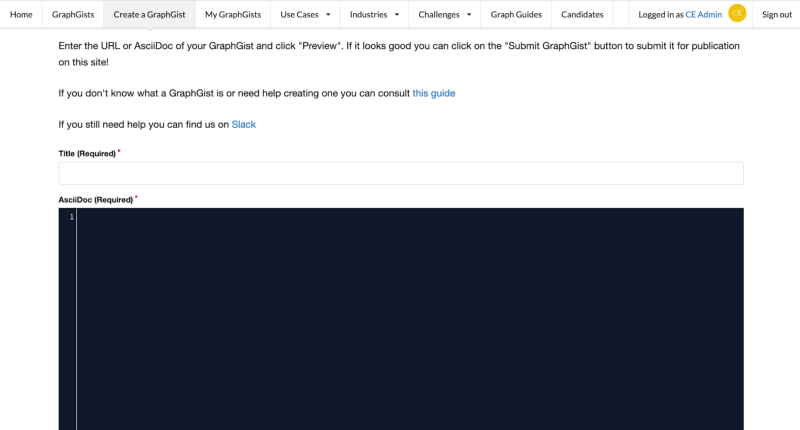
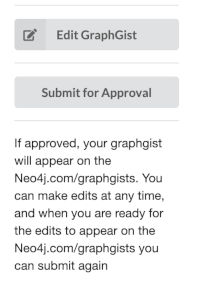
Administrator Users: Administer Content
Administrator users can:
- View and edit graph candidates
- Promote candidates to graph examples
- Assign graph examples to industries, use cases, and challenges
- Create and manage industries, use cases, and challenges
- Handle notifying admins there is new content to review and approve via automated emails
Administrators can edit the Graph Example’s metadata, including the summary, image, associated Use Cases, Industries, and Challenges.
After the administrator is happy with the content, the admin can approve the candidate, promoting it to be able to be seen and searched by the public.
The Code
The code for the CMS portal is all available on GitHub, feel free to use it for yourselves or to learn from it:
neo4j-contrib/graphgist-portal-v3
GRANDstack Implementation
Why Migrate to GRANDstack?
Although the Rails implementation was fine and had been serving us for five years, the portal would be more manageable by migrating to an actively supported stack (Javascript, GraphQL, React) and was due for a database upgrade. The old portal’s data was transferred into a fully managed 4.x Neo4j Aura database.
GRANDstack brings the combination of React, GraphQL, and Neo4j to the table. One can easily set up a new app with little to no previous experience with Cypher (the Neo4j query language), as GRANDstack takes care of GraphQL-to-Cypher translations through neo4j-graphql-js.
The GRANDstack starter allows developers to easily get started with:
npx create-grandstack-app
Which walks you through some config and even allows to infer the schema from the data in a database.
All you really need to get started is to define a simple data schema of GraphQL types. Once the schema is defined, you can manipulate all data via the auto-generated GraphQL API!
No migrations and no need to deal with exposing API methods manually with a lot of boilerplate code!
A GraphQL Query Request
Here is how a request coming from a GraphQL query is translated into Cypher and executed against the database to be returned to the caller.
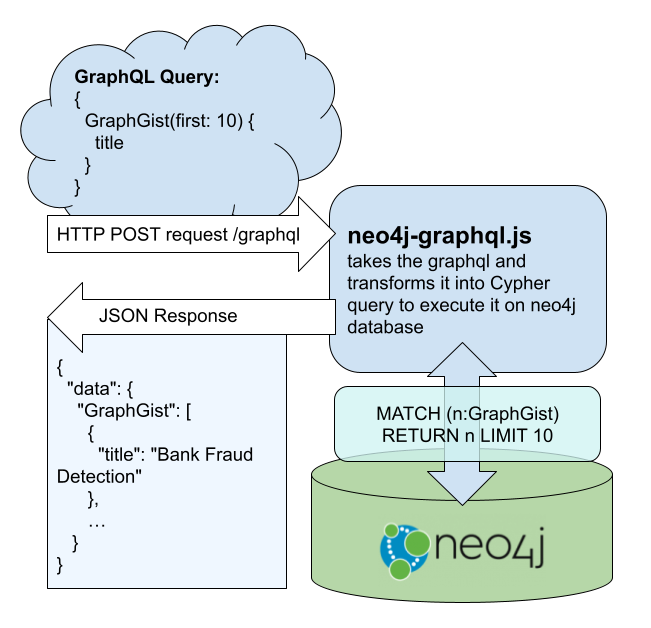
Implementation: Browse Graph Examples
Either by finding the portal via a search engine or being referred to it by a friend, anonymous users can browse, search, and view individual graph examples on the portal:
The schema type for the GraphGist entity shown on that view is quite large but some of that is due to legacy field management.
But as soon as you have the schema defined and made known to the library it generates all the enriched GraphQL types and API queries and mutations for you that you can explore and immediately e.g. in GraphiQL
View a List of Graph Examples, Filtered by Use Case
After viewing a single Graph Example, visitors may want to browse Graph Examples in the same Industry or Use Case. We could have done this with some of the advanced filter options, but it was easier to just use the @cypher directive to implement a custom query.
Again immediately usable in the API.
Here is the equivalent result as Nodes and Relationships in Neo4j Browser.
Rate Published Graph Examples
While browsing a Graph Example on the portal, a visitor might decide to give the item a star rating. If unauthenticated, the visitor will have to log in or create an account to use the rating feature.
Create Candidate Content
After browsing and rating existing Graph Examples, a user may decide to create their own content which is initially a “candidate. After the Graph Example Candidate is created, the Administrator users receive an email alerting them of the update, and decide whether or not to approve the content.
The type for the GraphGistCandidate adds a few more fields and relationships from Asset to allow for the content-management aspects of the publishing flow.
Here is a GraphGistCandidate pointing to the live version of an GraphGist with it’s Author, their User and the Industry.
GRANDStack Resources
This blog post was just a brief overview of a very utilitarian GRANDStack application. Feel encouraged to build your own GRANDStack application with the resources below, and stay on the lookout for the next version of GRANDStack.
- Fullstack GraphQL Applications with GRANDStack – Essential Excerpts
- Build Fullstack GraphQL Applications With Ease | GRANDstack
- Build a Neo4J & GraphQL API
About the Authors
Cristina and Alisson work at The SilverLogic, a software development company based in Boca Raton.
Alisson is a software engineer at The SilverLogic. Passionate about plants, he is the driving force behind Que Planta, a GraphQL-based social network for plants.
Register for NODES 2021 today and enjoy the talks from experienced graph developers.
Save My Spot
Building a GraphQL-Based CMS with the GRANDstack was originally published in Neo4j Developer Blog on Medium, where people are continuing the conversation by highlighting and responding to this story.