Now that you have data in your Neo4j database, you want to take it to the next level and create an application for your users — but don’t know where to start? Look no further. This starter kit is made for you!
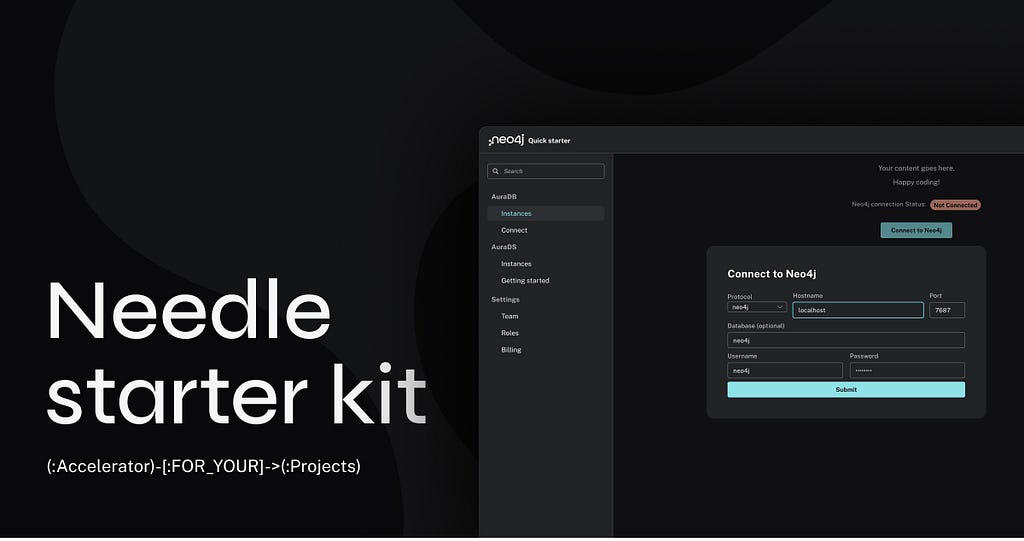
What is Neo4j Needle StarterKit?
The Neo4j Needle StarterKit is a template for developing applications aiming to reduce your development time and accelerate your Time To Value (TTV) ⏰. It uses the Neo4j Needle design system, providing a responsive and user-friendly interface for applications that interact with Neo4j databases.
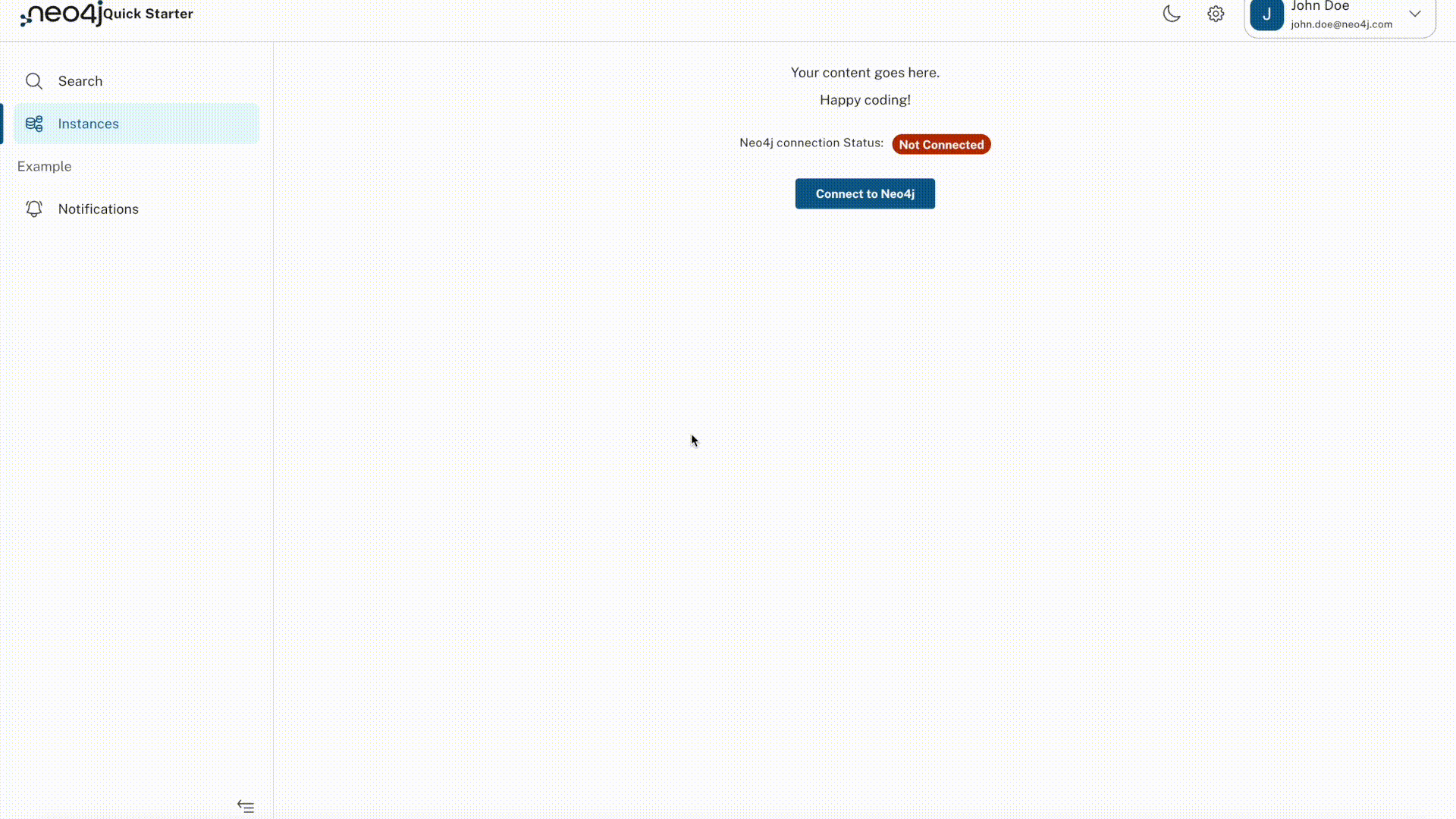
Key Features
- 🚀 Responsive: Adapts to different screen sizes for optimal user experience.
- 🌚 Dark/Light Mode Theme 🌞: Includes a theme wrapper to switch between light and dark modes without having to reinvent the wheel!
- ⚙️ Neo4j Integration: A simple example for connecting to a Neo4j database.
- 🔐 Neo4j Auto-connect: Automatically connects to the Neo4j database if the user has a session saved (using localStorage).
- 💻 Side Navigation: Offers a navigational sidebar for easy access to different parts of the application.
- 🛠️️ Modular approach: Facilitates easy customization.
Component Architecture
The StarterKit’s architecture is modular, with each component serving a specific purpose. The main components are:
- App: The root component that wraps everything within the ThemeWrapper.
- QuickStarter: Serves as the primary layout component, including the Header and PageLayout.
- Header: Contains the app bar with theme toggle and user information.
- PageLayout: Organizes the SideNav and the main Content.
- Content: The central area where the application’s main content is displayed.
- ConnectionModal: A modal dialog for connecting to a Neo4j database.
- Driver: Small utility class to connect to/disconnect from a Neo4j database.
How to Use the StarterKit
Installation
To get started, ensure you have a Neo4j DB up and running. The easiest way to get this done is by starting a free Neo4j instance on Neo4j Aura, Sandbox, or Desktop (see the additional resources section at the end of this article).
Then, all you need to do is clone the repository and install the dependencies:
npm i
npm run dev
Code Snippets
Here are some key components of the Starter kit:
App Component (App.tsx)
function App() {
return (
<ThemeWrapper>
<QuickStarter />
</ThemeWrapper>
);
}
The main App is pretty simple. We have our ThemeWrapper (which enables us to have the light/dark mode theme applied to every component of our app without having to apply it everywhere) and the QuickStarter component, which is our main app (composed of the Header and Layout components)
Light/Dark mode (Header.tsx)
<IconButton clean size="large" onClick={themeUtils.toggleColorMode}>
{theme.palette.mode === "dark" ? (
<span role="img" aria-label="sun">
<SunIconOutline />
</span>
) : (
<span role="img" aria-label="moon">
<MoonIconOutline />
</span>
)}
</IconButton>;
The selection of light/dark mode is done in the Header component through the toggle click that triggers the setMode in the ThemeWrapper, which then applies/injects the required classes based on the selected mode. You can then access the current mode in any child by using: theme.palette.mode
Driver (Driver.tsx)
export async function setDriver (connectionURI, username, password){
try{
driver = neo4j.driver(connectionURI, neo4j.auth.basic(username, password))
const serverInfo = await driver.getServerInfo()
localStorage.setItem("neo4j.connection", JSON.stringify({"uri":connectionURI, "user":username, "password":password}))
return true
} catch (err){
console.log(`Connection error\n${err}\nCause: ${err.cause}`)
return false
}
}
export async function disconnect (){
try{
driver.close();
return true;
} catch (err){
console.log(`Disconnection error\n${err}\nCause: ${err.cause}`)
return false
}
}
The Driver util is composed of 2 functions:
setDriver: Takes a Neo4j URI, username, and password to create a driver connection to Neo4j. If successful, save the connection details to the localStorage (for future auto-login) and return true. If the connection fails, return false and log an error with the details.
disconnect: Close the current driver or return an error.
Auto-login (Content.tsx)
useEffect(() => {
if (!init) {
let session = localStorage.getItem("neo4j.connection");
if (session) {
let neo4jConnection = JSON.parse(session);
setDriver(
neo4jConnection.uri,
neo4jConnection.user,
neo4jConnection.password
).then((isSuccessful: boolean) => {
setConnectionStatus(isSuccessful);
});
}
setInit(true);
}
});
The auto-login feature is done through a useEffect hook on the Content component. When the page is initialized, we check if there is a connection saved in the localStorage. If one is found, we use it to make a connection to the Neo4j DB. The connection status is then accessible through the state: connectionStatus, making it easy to separate the content you want to display if a Neo4j connection is made or not:
{
!connectionStatus ? (
<>Not connected content goes here</>
) : (
<>Connected content goes here</>
);
}
Connection (ConnectionModal.tsx)
function submitConnection() {
const connectionURI = selectedProtocol + "://" + hostname + ":" + port;
setDriver(connectionURI, username, password).then((isSuccessful) => {
setConnectionStatus(isSuccessful);
});
setOpenConnection(false);
}
The connection component is a simple modal that submits the Neo4j URI, username, and password entered by the user to the Driver util we saw earlier.
Conclusion
In conclusion, the Neo4j Needle StarterKit is an excellent starting point for developers looking to build applications with Neo4j. Its responsive design, theme flexibility, and modular architecture make it a versatile and user-friendly choice.
If you encounter issues or have any feedback on this starter kit, please feel free to:
- Reach out on our Neo4j Discord or our community forum.
- Create git issues or PR.
What Next?
Now that you’re familiar with the basics of the template, it’s time to explore the possibilities this opens for you. Here are some ideas and directions you can take as simple next steps:
- Run your first Cypher query through the driver with a callback function. When you receive the data, display it as a text or table.
- From the step above, let’s make it a bit more visual by using a visualization library and rendering your graph data in your app.
- Enhance the application security by adding an authentication mechanism.
Additional Resources
- GitHub – msenechal/neo4j-needle-starterkit: Starter Kit for building a Neo4j app using Neo4j Needle
- Neo4j Aura – Fully Managed Cloud Solution
- Home – Neo4j Sandbox
- Developer Tools
Needle StarterKit: The Ultimate Tool for Accelerating Your Graph App Projects was originally published in Neo4j Developer Blog on Medium, where people are continuing the conversation by highlighting and responding to this story.