Implementing Your First Model
About this module
At the end of this module, you will be able to:
-
Write Cypher code to implement a simple initial graph data model.
-
Confirm that the starter data is in the graph.
Domain used for this training
For this training we will work with a familiar domain, airplane flights. We are working in a familiar domain so that we can focus on implementation details, and not be distracted by wider concepts.
Specifically, we will use a dataset from the US Bureau of Transportation.
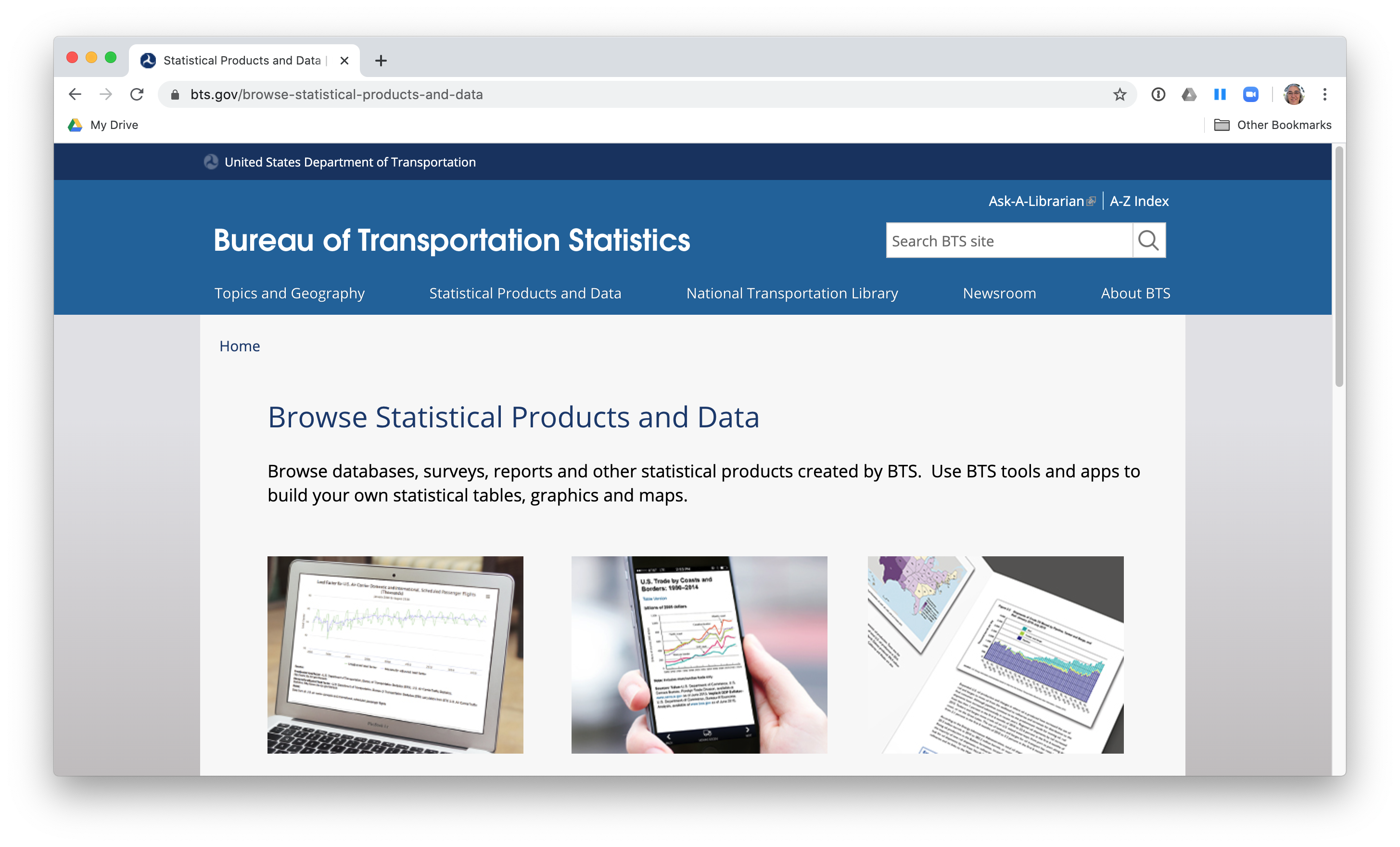
Application question for our model
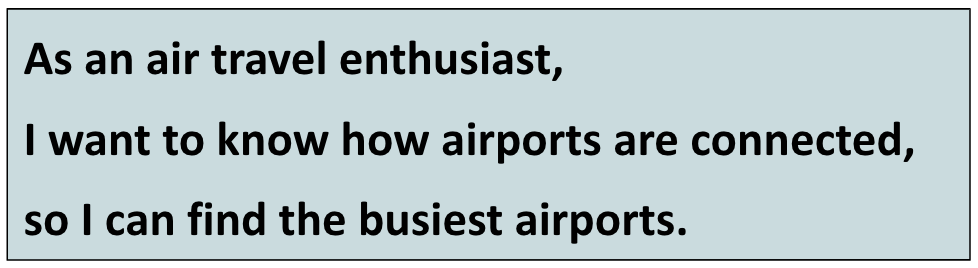
For this question:
-
What are the entities?
-
What are the connections between entities?
-
What properties are needed?
Working with the sample data
A best practice when creating a graph with Cypher is to use the MERGE
statement.
When creating nodes, you specify the properties in the MERGE
statement to ensure that nodes with the same property values are not duplicated in the graph.
For large graphs, the node properties used for the MERGE must have uniqueness constraints on them.
In the next exercise, Exercise 1, you will:
-
Create three Airport nodes and two CONNECTED_TO relationships per the initial data model by using the
MERGE
statement. -
Query the graph to show the newly-created nodes and relationships.
Creating the nodes and relationships using the MERGE
statement and hard-coding values is fine for small sample data, but once you start working with larger amounts of data, you will want to load the data (typically from CSV files).
Exercise 1: Getting started with the airport graph data model
Before you start this exercise you must:
-
Create a project in Neo4j Desktop, create a blank sandbox, or create a Neo4j Aura instance.
-
If using Neo4j Desktop, create a local 4.x database in the project and start it.
-
Open a Neo4j Browser window for the database.
In the query edit pane of Neo4j Browser, execute the browser command:
:play 4.0-neo4j-modeling-exercises
and follow the instructions for Exercise 1.
This exercise has 3 steps. Estimated time to complete: 15 minutes. |
Check your understanding
Question 1
What Cypher statement is a best practice for adding nodes and relationships to the graph?
Select the correct answer.
-
CREATE
-
ADD
-
MERGE
-
INSERT
Question 2
Given this code:
CREATE (:Person {name:"Joe"}
CREATE (:Person {name:"Jane"}
MERGE (:Person {name:"Bob"}
MERGE (:Person {name:"Joe"}
How many nodes are created in the graph?
Select the correct answer.
-
0
-
2
-
3
-
4
Question 3
Given this code:
MERGE (a:Airport {code:'LAS'})
MERGE (b:Airport {code:'LAX'})
MERGE (c:Airport {code:'ABQ'})
MERGE (a)-[:CONNECTED_TO {airline:'WN',flightNumber:'82',date:'2019-1-3',departure:'1715',arrival:'1820'}]->(b)
MERGE (a)-[:CONNECTED_TO {airline:'WN',flightNumber:'500',date:'2019-1-3',departure:'1445',arrival:'1710'}]->(c)
What is the implied entity for this domain?
Select the correct answer.
-
Airport
-
code
-
airline
-
CONNECTED_TO
Summary
You can now:
-
Write Cypher code to implement a simple initial graph data model.
-
Confirm that the starter data is in the graph.
Need help? Ask in the Neo4j Community