apoc.refactor.cloneSubgraph
Procedure
apoc.refactor.cloneSubgraph(nodes LIST<NODE>, rels LIST<RELATIONSHIP>, config MAP<STRING, ANY>)
- clones the given NODE
values with their labels and properties (optionally skipping any properties in the skipProperties
LIST<STRING>
via the config MAP
), and clones the given RELATIONSHIP
values.
If no RELATIONSHIP
values are provided, all existing RELATIONSHIP
values between the given NODE
values will be cloned.
Signature
apoc.refactor.cloneSubgraph(nodes :: LIST<NODE>, rels = [] :: LIST<RELATIONSHIP>, config = {} :: MAP) :: (input :: INTEGER, output :: NODE, error :: STRING)
Usage Examples
The examples in this section are based on the following sample graph:
CREATE (rootA:Root{name:'A'}),
(rootB:Root{name:'B'}),
(n1:Node{name:'node1', id:1}),
(n2:Node{name:'node2', id:2}),
(n3:Node{name:'node3', id:3}),
(n4:Node{name:'node4', id:4}),
(n5:Node{name:'node5', id:5}),
(n6:Node{name:'node6', id:6}),
(n7:Node{name:'node7', id:7}),
(n8:Node{name:'node8', id:8}),
(n9:Node{name:'node9', id:9}),
(n10:Node{name:'node10', id:10}),
(n11:Node{name:'node11', id:11}),
(n12:Node{name:'node12', id:12})
CREATE (rootA)-[:LINK]->(n1)-[:LINK]->(n2)-[:LINK]->(n3)-[:LINK]->(n4)
CREATE (n1)-[:LINK]->(n5)-[:LINK]->(n6)<-[:LINK]-(n7)
CREATE (n5)-[:LINK]->(n8)
CREATE (n5)-[:LINK]->(n9)-[:DIFFERENT_LINK]->(n10)
CREATE (rootB)-[:LINK]->(n11);
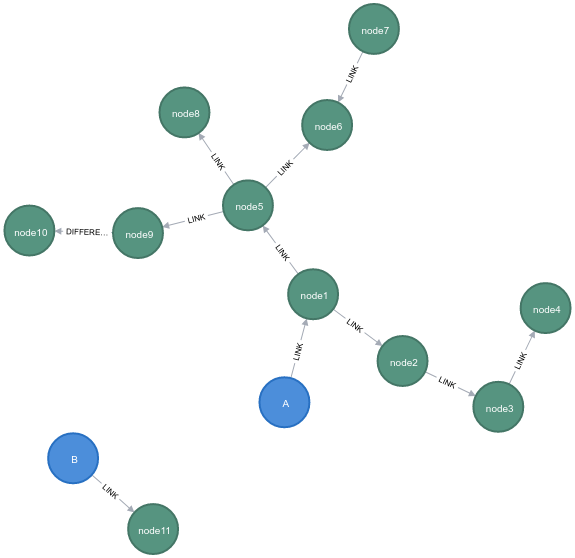
This procedure clones a subgraph defined by a list of nodes and a list of relationships. If relationships are not provided, all relationships between the given nodes will be cloned. This is useful when you want to ensure the cloned subgraph isn’t connected to the original nodes, or to nodes outside the subgraph.
We can get the nodes and relationships from the yielded output of apoc.path.subgraphAll, filtering to the relationship types in the call to that procedure.
The following query clones a subtree starting from rootA consisting of outgoing :LINK
relationships, and attaches that subgraph to rootB. rootB acts as a standin for rootA, which is not cloned:
MATCH (rootA:Root{name:'A'}),
(rootB:Root{name:'B'})
CALL apoc.path.subgraphAll(rootA, {relationshipFilter:'LINK>'})
YIELD nodes, relationships
CALL apoc.refactor.cloneSubgraph(
nodes,
[rel in relationships WHERE type(rel) = 'LINK'],
{ standinNodes:[[rootA, rootB]] })
YIELD input, output, error
RETURN input, output, error;
input | output | error |
---|---|---|
31378 |
(:Node {name: "node1", id: 1}) |
NULL |
31382 |
(:Node {name: "node5", id: 5}) |
NULL |
31379 |
(:Node {name: "node2", id: 2}) |
NULL |
31386 |
(:Node {name: "node9", id: 9}) |
NULL |
31383 |
(:Node {name: "node6", id: 6}) |
NULL |
31385 |
(:Node {name: "node8", id: 8}) |
NULL |
31380 |
(:Node {name: "node3", id: 3}) |
NULL |
31381 |
(:Node {name: "node4", id: 4}) |
NULL |
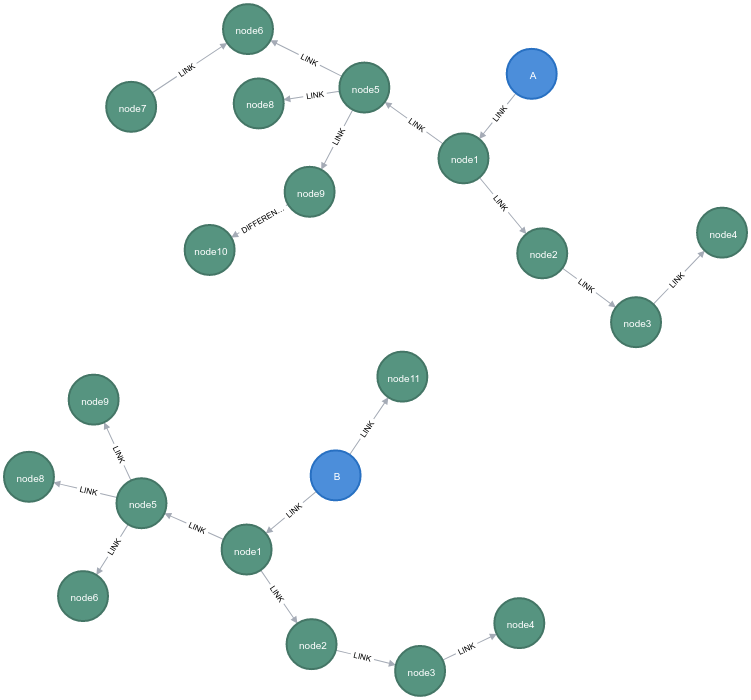