UUIDs
The library supports manual and automation generation of UUIDs, which can be stored as properties on nodes.
UUIDs are generated using the java randomUUID utility method, which generates a v4UUID.
UUID may be encoded into String with well-known hexadecimal presentation (32 characters, e.g. 1051af4f-b81d-4a76-8605-ecfb8ef703d5
) or Base64 (22 characters, e.g. vX8dM5XoSe2ldoc/QzMEyw
)
Manual UUIDs
Qualified Name | Type | Release |
---|---|---|
|
|
|
- create an UUID encoded with Base64 |
|
|
- convert between an UUID encoded with Base64 to HEX format |
|
|
- convert an UUID in HEX format to encoded with Base64 |
|
|
Usage Examples
The following generates a UUID
RETURN apoc.create.uuid() AS uuid;
uuid |
---|
"1051af4f-b81d-4a76-8605-ecfb8ef703d5" |
The following creates a Person
node, using a UUID as the merging key:
MERGE (p:Person {id: apoc.create.uuid()})
SET p.firstName = "Michael", p.surname = "Hunger"
RETURN p
p |
---|
{"firstName":"Michael","surname":"Hunger","id":"5530953d-b85e-4939-b37f-a79d54b770a3"} |
The following generates a new UUID encoded with Base64:
RETURN apoc.create.uuidBase64() as output;
Output |
---|
"vX8dM5XoSe2ldoc/QzMEyw" |
The following converts an UUID encoded with Base64 to HEX representation:
RETURN apoc.create.uuidHexToBase64("bd7f1d33-95e8-49ed-a576-873f433304cb") as output;
Output |
---|
"vX8dM5XoSe2ldoc/QzMEyw" |
The following converts an UUID encoded with Base64 to HEX representation:
RETURN apoc.create.uuidBase64ToHex("vX8dM5XoSe2ldoc/QzMEyw") as output;
Output |
---|
"bd7f1d33-95e8-49ed-a576-873f433304cb" |
Automatic UUIDs
There are also procedures that handle automatic adding of UUID properties, via the UUID Handler Lifecycle. The UUID handler is a transaction event handler that automatically adds the UUID property to a provided label and for the provided property name. Please check the following documentation to an in-depth description.
All these procedures (except the list and show ones) are intended to be executed in the system database,
therefore they have to be used executed by opening a system database session. There are several ways of doing this:
- when using cypher-shell or Neo4j Browser, one can prefix their Cypher query with Moreover, they accept as first parameter the name of the database towards which we want to install/update/remove the automatic UUIDs. Through this implementation, we can use these procedures in a cluster environment, by leveraging the cluster routing mechanism. |
Installing, updating or removing an automatic UUID is an eventually consistent operation.
Therefore, they are not immediately added/updated/removed,
but they have a refresh rate handled by the Apoc configuration |
Enable apoc.uuid.enabled=true
or apoc.uuid.enabled.[DATABASE_NAME]=true
in $NEO4J_HOME/config/apoc.conf
first.
Configuration value apoc.uuid.format
let you choose between different UUID encoding methods: hex
(default option) or base64
.
Qualified Name | Type | Release |
---|---|---|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
UUID Examples
Create an Automatic UUID
This examples assume that we are on the neo4j
database,
and we want to create the automatic UUIDs in that database.
Add the uuid
:
CALL apoc.uuid.setup('Person')
YIELD label, installed, properties
RETURN label, installed, properties
Note that the apoc.uuid.setup
, as well as the apoc.uuid.drop
, apoc.uuid.dropAll
,
have to be executed in the system database.
The result is:
label | installed | properties |
---|---|---|
"Person" |
true |
|
When an apoc.uuid.setup
procedure is executed,
a query to create a constraint, if not exists, is automatically executed (also in this case, after a time defined by the apoc.uuid.refresh
config):
CREATE CONSTRAINT IF NOT EXISTS FOR (n:<label>) REQUIRE (n.<uuidProperty>) IS UNIQUE
It is then possible to execute the following query, after a time defined by the configuration apoc.uuid.refresh
:
CREATE (n:Person {name: 'Daniel'})-[:Work]->(:Company {name: 'Neo4j'})
and the result will be a node :Person
with 2 properties:
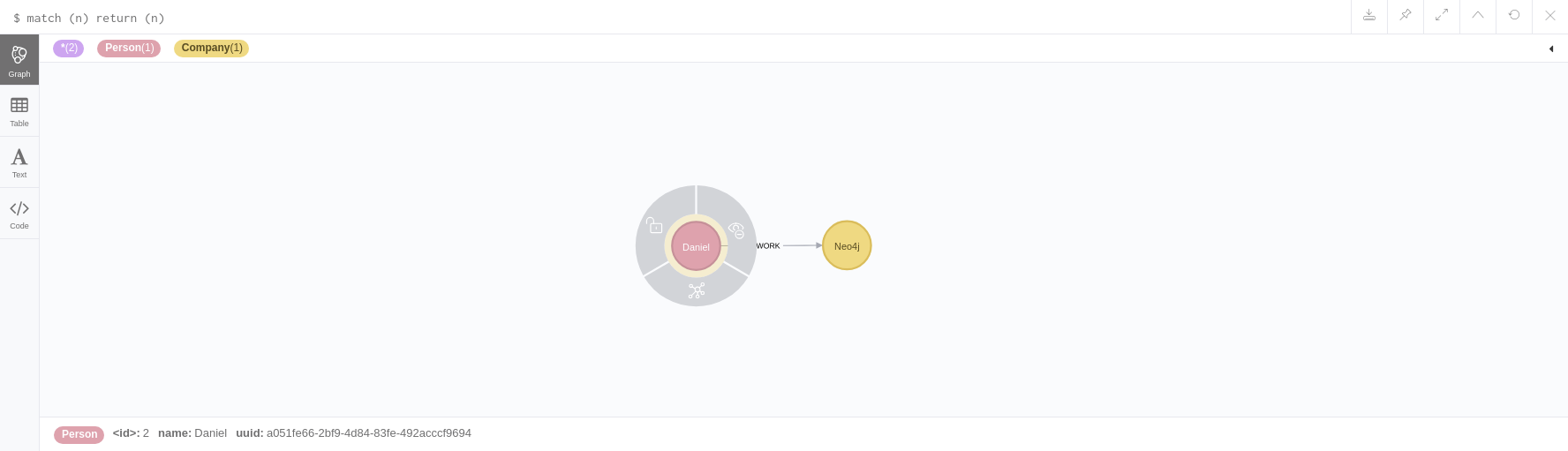
If the default configuration addToExistingNodes: true
is chosen,
in background (via the apoc.periodic.iterate
procedure) all existing nodes will also be populated with a property with uuid values.
and, when the execution is finished,
a log with the query result like the following will be printed:
Result of batch computation obtained from existing nodes for UUID handler with label `MyLabel`:
{failedParams={}, committedOperations=1, batch={total=10, committed=10, failed=0, errors={}}, wasTerminated=false, batches=1, timeTaken=0, retries=0, errorMessages={}, total=1, operations={total=10, committed=10, failed=0, errors={}}, failedOperations=0, updateStatistics={nodesDeleted=0, labelsAdded=0, relationshipsCreated=0, nodesCreated=0, propertiesSet=1, relationshipsDeleted=0, labelsRemoved=0}, failedBatches=0}
List of Automatic UUIDs
It is possible to return the full list of automatic UUIDS in a database. For example, if the UUID in the following query is created:
CALL apoc.uuid.setup('TestShow')
It is then possible to run (also in this case, after a time defined by the configuration apoc.uuid.refresh
):
CALL apoc.uuid.show()
label | installed | properties | "Person" |
---|
Please note that, since the auto-UUID operations are eventually consistent (based on the |
Remove Automatic UUIDs
CALL apoc.uuid.drop('Person')
YIELD label, installed, properties
RETURN label, installed, properties
The result is:
label | installed | properties |
---|---|---|
"Person" |
false |
{uuidProperty → "uuid", addToExistingNodes → true} |
You can also remove all the uuid installed call the procedure as:
CALL apoc.uuid.dropAll()
YIELD label, installed, properties
RETURN label, installed, properties
The result is:
label | installed | properties |
---|---|---|
"Person" |
false |
{uuidProperty → "uuid", addToExistingNodes → true} |
Export metadata
To import uuids in another database (for example after a |