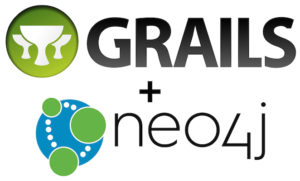
[As community content, this post reflects the views and opinions of the particular author and does not necessarily reflect the official stance of Neo4j.]
Mapping object models to the Neo4j graph in languages such as Java and Groovy may be immensely convenient when developing your application, but has the danger of obfuscating the power of Neo4j.With the recently released GORM for Neo4j 6.1 at OCI, the home of the core Grails team, we built a tool that takes advantage of the native features exposed by the Neo4j Bolt Java Driver.
Get Started
If you want to get started immediately, we’ve provided a detailed tutorial that walks through all the features that GORM provides for Neo4j.
You’ll use GORM and Grails to develop a simple web application for the built-in movie database. The guide covers the backend with domain model and services, which provide REST endpoints to a JavaScript UI.
A repository with the resulting Grails application is available here.
If you’d rather use Spring Boot with Web-MVC, GORM provides you the same benefits. Here is a repository for an example Spring Boot application that achieves the same thing.
But now let’s look at GORM for Neo4j in some more detail.
Model Nodes, Relationships and Paths
GORM for Neo4j allows you to model not just nodes, but also to map Groovy classes to relationships.
Combine all of this with the supporting features that you would expect from an object mapping tool, such as:
- Dirty checking and optimized updates
- Batch writes with
UNWIND
- Transaction management
- Customizable ID generation
- Optimistic locking
- Lazy loading
@Entity
:import grails.gorm.annotation.* @Entity class Movie { String title String tagline int released }
Relationships can be modelled by using the
grails.neo4j.Relationship
trait:import grails.neo4j.Relationship @Entity class CastMember implements Relationship{ List roles = [] }
These can then be queried either natively or using GORM’s existing rich query API.
Later you can use more advanced GORM features like constraints or has-many mappings.
class Movie { String title String tagline int released static hasMany = [cast: CastMember] static constraints = { released min:1900 title blank:false } }
Groovy and Neo4j: A Natural Fit
With Neo4j being a schema-less database, Groovy’s ability to simplify dynamic code (whilst still providing support for static compilation) really shines.
You can of course statically declare the properties of your domain class, but if you need to assign dynamic properties to individual objects, that is not a problem with Groovy.
For example, you can read and write dynamic properties using Groovy’s subscript operator, thus not limiting yourself to a statically defined schema:
Movie movie = Movie.findByTitle("The Matrix") movie["rating"] = 5 println "The movie ${movie} is rated ${movie['rating']}"
Harness the Power of Cypher
GORM for Neo4j provides many awesome ways to build queries, though none of these impacts your ability to use the native power of Cypher.
You can use Cypher for all your queries, or just to solve specific problems, such as finding the shortest path.
You can even get GORM to write your Cypher query logic for you, using GORM Data Services!
For example the following interface uses the
@Cypher
annotation to automatically implement a method called updatePerson
for you.import grails.gorm.services.Service import grails.neo4j.services.Cypher @Service(Person) interface PersonService { @Cypher("""MATCH ${Person p} WHERE ${p.name} = ${name} SET ${p.age} = ${age}""") void updatePerson(String name, int age) }
NOTE: Compile time checking is applied against the classes and property references including within the Cypher query!
Built for the Real World
Our preference at OCI is to always build solutions based on real-world experiences, and with GORM for Neo4j that hasn’t changed.
We worked together with Brinqa – a leading provider of unified risk management – to build an object mapping solution for Neo4j that addresses their real-world challenges.
Example Application and Tutorial
Curious to know more? We have prepared a detailed tutorial based on the original Neo4j movies data model that demonstrates building an application with Grails, GORM 6.1 and Neo4j. Please check it out and let us know if you have any suggestions for improvement.
About OCI
We write some of the world’s most powerful software solutions, are deeply committed to open source, and have trained over 50,000 developers. Our Grails Team includes the founders of the framework, along with subject matter experts from around the globe.
Engaging our team enables quicker project upgrades, improving overall speed-to-market. Please contact us today to discuss your next Grails project.
Sign Me Up