Integrate Neo4j With Symfony: A Step-by-Step Guide
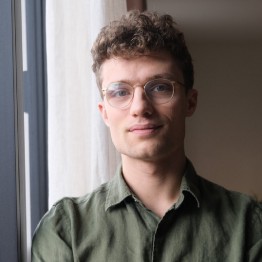
CEO of Nagels IT Consulting
5 min read
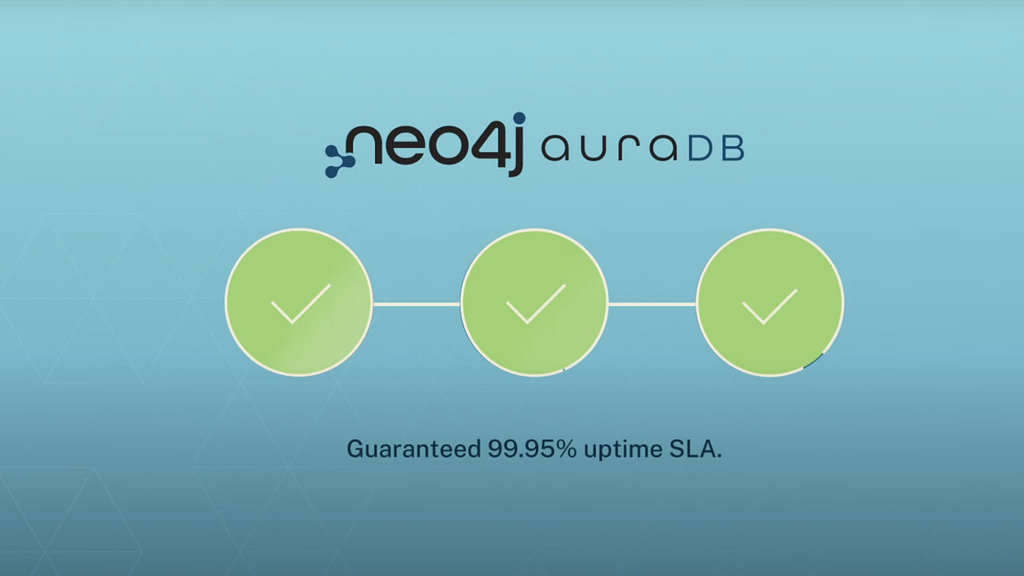
Celebrating the official release of the Neo4j Symfony bundle, we’re launching a series of four blogs about integrating Neo4j with Symfony. Here, we’ll guide you through the setup and configuration process, starting with the basics and gradually diving into more advanced techniques and features in each subsequent post. By the end, you’ll have a comprehensive understanding of using Neo4j’s graph database with Symfony for powerful data-driven applications.
Symfony is a robust PHP framework known for its flexibility in building web applications. Combined with Neo4j, a graph database designed for handling complex relationships and connected data, it becomes a powerful solution for advanced data management. We’ll guide you step by step through integrating Neo4j with Symfony, focusing on using Neo4j Aura, the cloud version of Neo4j.
By the end of this guide, you will have a Symfony application connected to Neo4j and be able to run basic queries to interact with the database.
We’ll cover:
- Installing Neo4j in Symfony using Composer
- Setting up Neo4j Aura for cloud-based Neo4j databases
- Running queries with Symfony’s structure
Prerequisites
Before we dive into the process, make sure you have the following:
- A supported version of Symfony (version 5.4, 6.x, or 7.x at this time of writing)
- PHP (version 8.1 or higher)
- Composer installed for dependency management
- A Neo4j Aura instance (you can sign up for one for free through Neo4j Aura)
Step 1: Install Symfony and Set Up a New Project
Let’s set up a Symfony project. You can easily do this using the Symfony CLI.
Install the Symfony CLI if you haven’t done so by following the official documentation.
Create a new Symfony project by running the following command:
symfony new project-name --webapp
cd project-name
These commands will create a new Symfony project with enabled web application features and enter into the newly created project.
Note: While the web features are optional when using Neo4j integration, we’ll use some to demonstrate their interaction in a real-world scenario.
Step 2: Install the Neo4j Bundle With Composer
Before we set up the connection, let’s install the required Neo4j PHP client. This client allows Symfony to communicate seamlessly with the Neo4j database.
Open your terminal inside your Symfony project folder and run the following command:
composer require neo4j/neo4j-bundle
Thanks to an updated Symfony recipe, installing it with recipes enabled (the default behavior in Symfony) will automatically configure the driver for you! Symfony uses the environment variable NEO4J_DEFAULT_CONNECTION_DSNto configure the Neo4j bundle. (Thanks, Akshat, for getting this done!)
The next blog post in this series will look at how to configure the bundle to deal with more complex connectivity scenarios, including high availability.
Step 3: Configure the Connection URI
Speaking of DSNs, let’s set up a free Neo4j Aura instance to ensure that we have one to provide in our application.
Creating a Neo4j instance is seamless. Just follow the steps in the Neo4j Aura Quick Start Guide.
⚠️ Careful! The password is shared only once during the setup process, so make sure to store it in a safe place when you see it.
A connection URI looks like this:
neo4j+s://xxxxx.databases.neo4j.io
In your .env file at the root of your project, there will be something like this:
###> neo4j/neo4j-bundle ###
NEO4J_DEFAULT_CONNECTION_DSN=neo4j://<my-username>:<my-password>@localhost
###< neo4j/neo4j-bundle ###
The bundle recipe autogenerates this section. If it isn’t there, you can add it yourself.
The easiest way to complete the connectivity is to add the username and password to the connection URI in this format and enable SSL, a prerequisite for working with Neo4j Aura:
###> neo4j/neo4j-bundle ###
NEO4J_DEFAULT_CONNECTION_DSN=neo4j+s://neo4j:<my-password>@<my-instance>.databases.neo4j.io
###< neo4j/neo4j-bundle ###
⚠️ Be sure to change the password and instance address so it works!
Step 4: Testing the Connection
Now that your database connection is set up, let’s test it by creating a simple Symfony controller that will interact with the Neo4j database.
Generate a new controller by running the following command:
symfony console make:controller Neo4jTestController
Open the newly created Neo4jTestController.php file (located in src/Controller) and modify it to inject the Neo4j client, then run a basic query:
<?php
namespace AppController;
use LaudisNeo4jContractsSessionInterface;
use SymfonyBundleFrameworkBundleControllerAbstractController;
use SymfonyComponentDependencyInjectionAttributeAutowire;
use SymfonyComponentHttpFoundationResponse;
use SymfonyComponentRoutingAttributeRoute;
final class Neo4jTestController extends AbstractController
{
public function __construct(
#[Autowire(service: 'neo4j.session')]
private readonly SessionInterface $session
)
{
}
#[Route('/neo4j/test', name: 'app_neo4j_test')]
public function index(): Response
{
$query = 'MATCH (n) RETURN count(n) AS nodeCount';
$result = $this->session->run($query);
$nodeCount = $result->first()->get('nodeCount');
return new Response("Node count in the database: " . $nodeCount);
}
}
Start your server using the Symfony console:
symfony server:start
You can now navigate to http://localhost:8000/neo4j/test to view the node count!
Step 5: Creating and Finding Nodes
Let’s continue our story and create some nodes to show how easy it is to add new Neo4j features to our controller.
Create a node:
#[Route('/neo4j/create', name: 'app_neo4j_create')]
public function createNode(Request $request): Response
{
$name = $request->get('name', 'Pratiksha');
// Creating a new node person, with the property key "name"
// and user provided value or Neil by default
$result = $this->session->run(
'CREATE (n:Person {name: $name})',
['name' => $name])
);
return new Response('Node created successfully');
}
List the nodes:
#[Route('/neo4j/find', name: 'app_neo4j_find')]
public function findNode(): Response
{
$result = $this->session->run('MATCH (n:Person) RETURN n.name AS name');
$names = [];
foreach ($result as $record) {
$names[] = $record->get('name');
}
if (count($names) > 0) {
return new Response('Found nodes: ' . implode(', ', $names));
}
return new Response('No nodes found');
}
Note: This is, of course, a rudimentary way of managing CRUD operations. It only shows off the possibilities of the Neo4j bundle and the underlying driver.
Summary
We’ve walked through setting up a Symfony project, connecting it to a Neo4j AuraDB, and running basic queries. You now have a working foundation for building more complex applications using the power of graph databases in Symfony.
With Neo4j, you can efficiently manage relationships and connected data, allowing you to build more dynamic and data-driven applications. Happy coding!
Special Thanks
- Major props to Nabeel Parkar for picking up the development and maintenance of the Neo4j bundle.
- Thank you, Akshat Srivasta, for creating the bulk of the blog post and your first open source PR.
- Thank you, Michal Štefaňák, for relentlessly working on implementing the Bolt protocol in PHP. Your work has proven to be invaluable.
Integrate Neo4j With Symfony: A Step-by-Step Guide was originally published in Neo4j Developer Blog on Medium, where people are continuing the conversation by highlighting and responding to this story.